Manual instrumentation of Go applications with OpenTelemetry
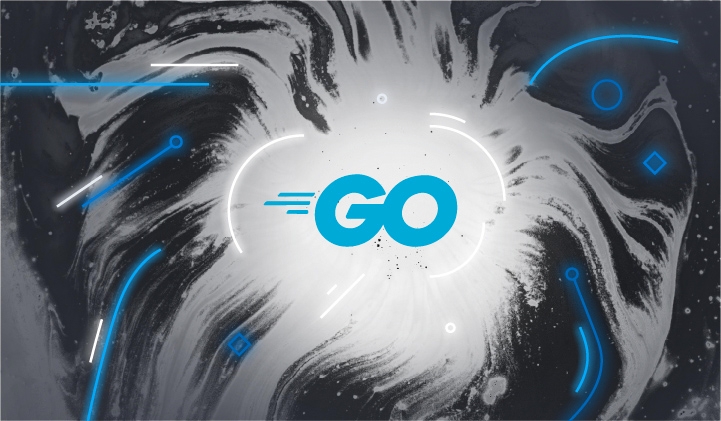
DevOps and SRE teams are transforming the process of software development. While DevOps engineers focus on efficient software applications and service delivery, SRE teams are key to ensuring reliability, scalability, and performance. These teams must rely on a full-stack observability solution that allows them to manage and monitor systems and ensure issues are resolved before they impact the business.
Observability across the entire stack of modern distributed applications requires data collection, processing, and correlation often in the form of dashboards. Ingesting all system data requires installing agents across stacks, frameworks, and providers — a process that can be challenging and time-consuming for teams who have to deal with version changes, compatibility issues, and proprietary code that doesn't scale as systems change.
Thanks to OpenTelemetry (OTel), DevOps and SRE teams now have a standard way to collect and send data that doesn't rely on proprietary code and have a large support community reducing vendor lock-in.
In this blog post, we will show you how to manually instrument Go applications using OpenTelemetry. This approach is slightly more complex than using auto-instrumentation
In a previous blog, we also reviewed how to use the OpenTelemetry demo and connect it to Elastic®, as well as some of Elastic’s capabilities with OpenTelemetry. In this blog, we will use an alternative demo application, which helps highlight manual instrumentation in a simple way.
Finally, we will discuss how Elastic supports mixed-mode applications, which run with Elastic and OpenTelemetry agents. The beauty of this is that there is no need for the otel-collector! This setup enables you to slowly and easily migrate an application to OTel with Elastic according to a timeline that best fits your business.
Application, prerequisites, and config
The application that we use for this blog is called Elastiflix, a movie streaming application. It consists of several micro-services written in .NET, NodeJS, Go, and Python.
Before we instrument our sample application, we will first need to understand how Elastic can receive the telemetry data.
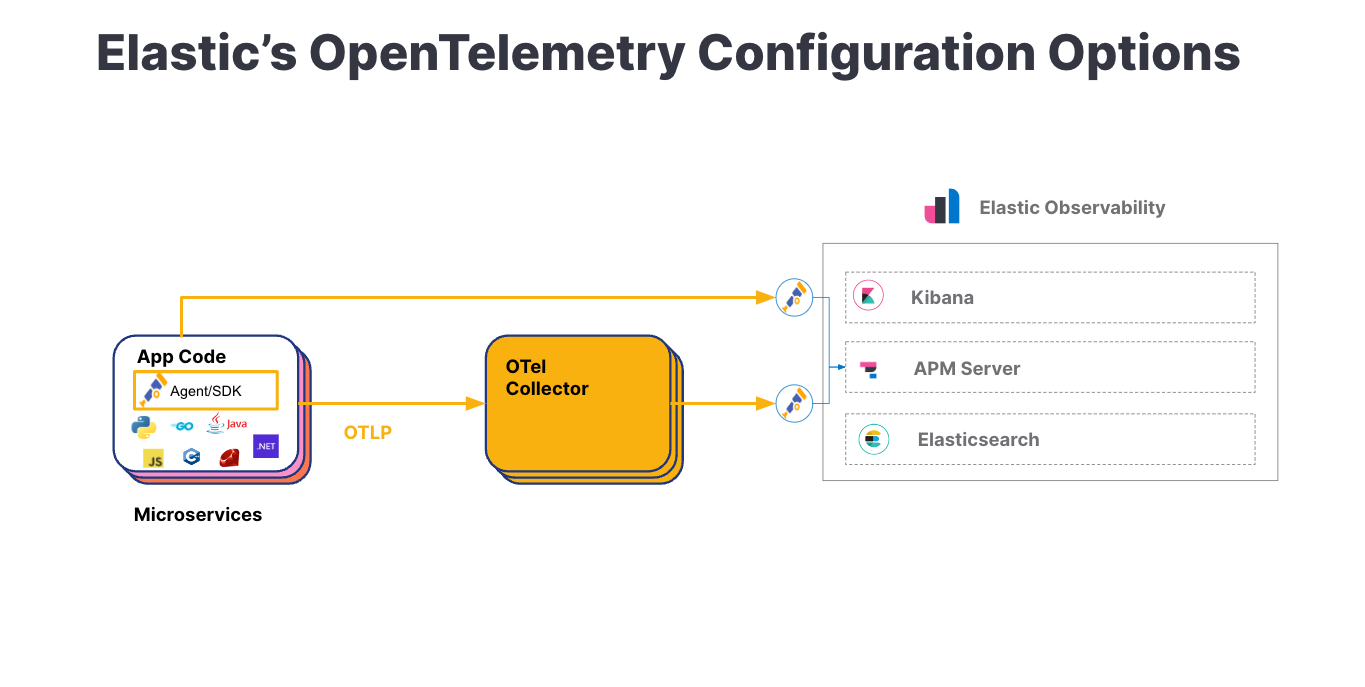
All of Elastic Observability’s APM capabilities are available with OTel data. Some of these include:
- Service maps
- Service details (latency, throughput, failed transactions)
- Dependencies between services, distributed tracing
- Transactions (traces)
- Machine learning (ML) correlations
- Log correlation
In addition to Elastic’s APM and a unified view of the telemetry data, you will also be able to use Elastic’s powerful machine learning capabilities to reduce the analysis, and alerting to help reduce MTTR.
Prerequisites
- An Elastic Cloud account — sign up now
- A clone of the Elastiflix demo application, or your own Go application
- Basic understanding of Docker — potentially install Docker Desktop
- Basic understanding of Go
View the example source code
The full source code including the Dockerfile used in this blog can be found on GitHub. The repository also contains the same application without instrumentation. This allows you to compare each file and see the differences.
Before we begin, let’s look at the non-instrumented code first.
This is our simple go application that can receive a GET request. Note that the code shown here is a slightly abbreviated version.
package main
import (
"log"
"net/http"
"os"
"time"
"github.com/go-redis/redis/v8"
"github.com/sirupsen/logrus"
"github.com/gin-gonic/gin"
"strconv"
"math/rand"
)
var logger = &logrus.Logger{
Out: os.Stderr,
Hooks: make(logrus.LevelHooks),
Level: logrus.InfoLevel,
Formatter: &logrus.JSONFormatter{
FieldMap: logrus.FieldMap{
logrus.FieldKeyTime: "@timestamp",
logrus.FieldKeyLevel: "log.level",
logrus.FieldKeyMsg: "message",
logrus.FieldKeyFunc: "function.name", // non-ECS
},
TimestampFormat: time.RFC3339Nano,
},
}
func main() {
delayTime, _ := strconv.Atoi(os.Getenv("TOGGLE_SERVICE_DELAY"))
redisHost := os.Getenv("REDIS_HOST")
if redisHost == "" {
redisHost = "localhost"
}
redisPort := os.Getenv("REDIS_PORT")
if redisPort == "" {
redisPort = "6379"
}
applicationPort := os.Getenv("APPLICATION_PORT")
if applicationPort == "" {
applicationPort = "5000"
}
// Initialize Redis client
rdb := redis.NewClient(&redis.Options{
Addr: redisHost + ":" + redisPort,
Password: "",
DB: 0,
})
// Initialize router
r := gin.New()
r.Use(logrusMiddleware)
r.GET("/favorites", func(c *gin.Context) {
// artificial sleep for delayTime
time.Sleep(time.Duration(delayTime) * time.Millisecond)
userID := c.Query("user_id")
contextLogger(c).Infof("Getting favorites for user %q", userID)
favorites, err := rdb.SMembers(c.Request.Context(), userID).Result()
if err != nil {
contextLogger(c).Error("Failed to get favorites for user %q", userID)
c.String(http.StatusInternalServerError, "Failed to get favorites")
return
}
contextLogger(c).Infof("User %q has favorites %q", userID, favorites)
c.JSON(http.StatusOK, gin.H{
"favorites": favorites,
})
})
// Start server
logger.Infof("App startup")
log.Fatal(http.ListenAndServe(":"+applicationPort, r))
logger.Infof("App stopped")
}
Step-by-step guide
Step 0. Log in to your Elastic Cloud account
This blog assumes you have an Elastic Cloud account — if not, follow the instructions to get started on Elastic Cloud.
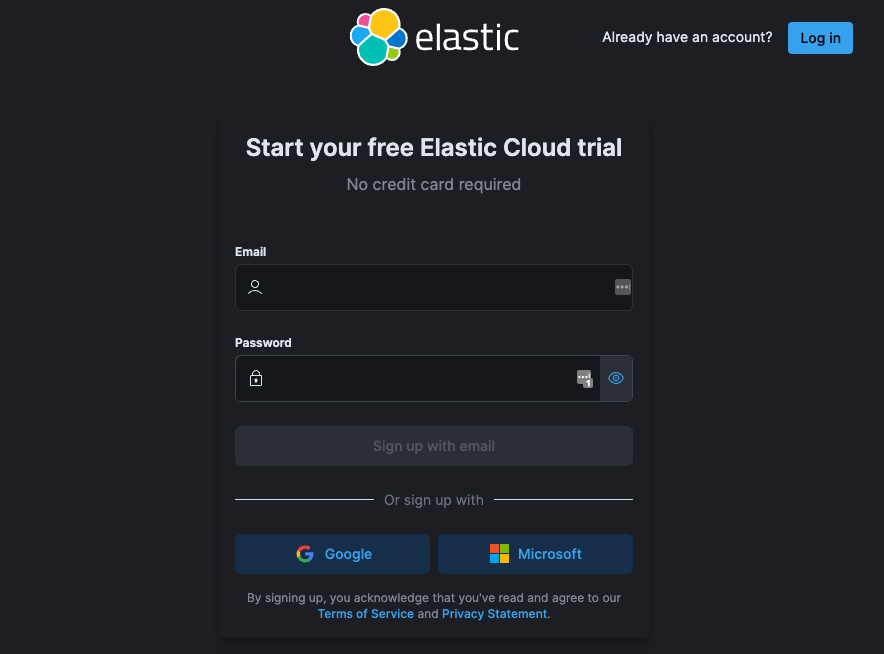
Step 1. Install and initialize OpenTelemetry
As a first step, we’ll need to add some additional packages to our application.
import (
"github.com/go-redis/redis/extra/redisotel/v8"
"go.opentelemetry.io/otel"
"go.opentelemetry.io/otel/attribute"
"go.opentelemetry.io/otel/exporters/otlp/otlptrace"
"go.opentelemetry.io/otel/exporters/otlp/otlptrace/otlptracegrpc"
"go.opentelemetry.io/otel/propagation"
"google.golang.org/grpc/credentials"
"crypto/tls"
sdktrace "go.opentelemetry.io/otel/sdk/trace"
"go.opentelemetry.io/contrib/instrumentation/github.com/gin-gonic/gin/otelgin"
"go.opentelemetry.io/otel/trace"
"go.opentelemetry.io/otel/codes"
)
This code imports necessary OpenTelemetry packages, including those for tracing, exporting, and instrumenting specific libraries like Redis.
Next we read the "OTEL_EXPORTER_OTLP_ENDPOINT" variable and initialize the exporter.
var (
collectorURL = os.Getenv("OTEL_EXPORTER_OTLP_ENDPOINT")
)
var tracer trace.Tracer
func initTracer() func(context.Context) error {
tracer = otel.Tracer("go-favorite-otel-manual")
// remove https:// from the collector URL if it exists
collectorURL = strings.Replace(collectorURL, "https://", "", 1)
secretToken := os.Getenv("ELASTIC_APM_SECRET_TOKEN")
if secretToken == "" {
log.Fatal("ELASTIC_APM_SECRET_TOKEN is required")
}
secureOption := otlptracegrpc.WithInsecure()
exporter, err := otlptrace.New(
context.Background(),
otlptracegrpc.NewClient(
secureOption,
otlptracegrpc.WithEndpoint(collectorURL),
otlptracegrpc.WithHeaders(map[string]string{
"Authorization": "Bearer " + secretToken,
}),
otlptracegrpc.WithTLSCredentials(credentials.NewTLS(&tls.Config{})),
),
)
if err != nil {
log.Fatal(err)
}
otel.SetTracerProvider(
sdktrace.NewTracerProvider(
sdktrace.WithSampler(sdktrace.AlwaysSample()),
sdktrace.WithBatcher(exporter),
),
)
otel.SetTextMapPropagator(
propagation.NewCompositeTextMapPropagator(
propagation.Baggage{},
propagation.TraceContext{},
),
)
return exporter.Shutdown
}
For instrumenting connections to Redis, we will add a tracing hook to it, and in order to instrument Gin, we will add the OTel middleware. This will automatically capture all interactions with our application, since Gin will be fully instrumented. In addition, all outgoing connections to Redis will also be instrumented.
// Initialize Redis client
rdb := redis.NewClient(&redis.Options{
Addr: redisHost + ":" + redisPort,
Password: "",
DB: 0,
})
rdb.AddHook(redisotel.NewTracingHook())
// Initialize router
r := gin.New()
r.Use(logrusMiddleware)
r.Use(otelgin.Middleware("go-favorite-otel-manual"))
Adding custom spans
Now that we have everything added and initialized, we can add custom spans.
If we want to have additional instrumentation for a part of our app, we simply start a custom span and then defer ending the span.
// start otel span
ctx := c.Request.Context()
ctx, span := tracer.Start(ctx, "add_favorite_movies")
defer span.End()
For comparison, this is the instrumented code of our sample application. You can find the full source code in GitHub.
package main
import (
"log"
"net/http"
"os"
"time"
"context"
"github.com/go-redis/redis/v8"
"github.com/go-redis/redis/extra/redisotel/v8"
"github.com/sirupsen/logrus"
"github.com/gin-gonic/gin"
"go.opentelemetry.io/otel"
"go.opentelemetry.io/otel/attribute"
"go.opentelemetry.io/otel/exporters/otlp/otlptrace"
"go.opentelemetry.io/otel/exporters/otlp/otlptrace/otlptracegrpc"
"go.opentelemetry.io/otel/propagation"
"google.golang.org/grpc/credentials"
"crypto/tls"
sdktrace "go.opentelemetry.io/otel/sdk/trace"
"go.opentelemetry.io/contrib/instrumentation/github.com/gin-gonic/gin/otelgin"
"go.opentelemetry.io/otel/trace"
"strings"
"strconv"
"math/rand"
"go.opentelemetry.io/otel/codes"
)
var tracer trace.Tracer
func initTracer() func(context.Context) error {
tracer = otel.Tracer("go-favorite-otel-manual")
collectorURL = strings.Replace(collectorURL, "https://", "", 1)
secureOption := otlptracegrpc.WithInsecure()
// split otlpHeaders by comma and convert to map
headers := make(map[string]string)
for _, header := range strings.Split(otlpHeaders, ",") {
headerParts := strings.Split(header, "=")
if len(headerParts) == 2 {
headers[headerParts[0]] = headerParts[1]
}
}
exporter, err := otlptrace.New(
context.Background(),
otlptracegrpc.NewClient(
secureOption,
otlptracegrpc.WithEndpoint(collectorURL),
otlptracegrpc.WithHeaders(headers),
otlptracegrpc.WithTLSCredentials(credentials.NewTLS(&tls.Config{})),
),
)
if err != nil {
log.Fatal(err)
}
otel.SetTracerProvider(
sdktrace.NewTracerProvider(
sdktrace.WithSampler(sdktrace.AlwaysSample()),
sdktrace.WithBatcher(exporter),
//sdktrace.WithResource(resources),
),
)
otel.SetTextMapPropagator(
propagation.NewCompositeTextMapPropagator(
propagation.Baggage{},
propagation.TraceContext{},
),
)
return exporter.Shutdown
}
var (
collectorURL = os.Getenv("OTEL_EXPORTER_OTLP_ENDPOINT")
otlpHeaders = os.Getenv("OTEL_EXPORTER_OTLP_HEADERS")
)
var logger = &logrus.Logger{
Out: os.Stderr,
Hooks: make(logrus.LevelHooks),
Level: logrus.InfoLevel,
Formatter: &logrus.JSONFormatter{
FieldMap: logrus.FieldMap{
logrus.FieldKeyTime: "@timestamp",
logrus.FieldKeyLevel: "log.level",
logrus.FieldKeyMsg: "message",
logrus.FieldKeyFunc: "function.name", // non-ECS
},
TimestampFormat: time.RFC3339Nano,
},
}
func main() {
cleanup := initTracer()
defer cleanup(context.Background())
redisHost := os.Getenv("REDIS_HOST")
if redisHost == "" {
redisHost = "localhost"
}
redisPort := os.Getenv("REDIS_PORT")
if redisPort == "" {
redisPort = "6379"
}
applicationPort := os.Getenv("APPLICATION_PORT")
if applicationPort == "" {
applicationPort = "5000"
}
// Initialize Redis client
rdb := redis.NewClient(&redis.Options{
Addr: redisHost + ":" + redisPort,
Password: "",
DB: 0,
})
rdb.AddHook(redisotel.NewTracingHook())
// Initialize router
r := gin.New()
r.Use(logrusMiddleware)
r.Use(otelgin.Middleware("go-favorite-otel-manual"))
// Define routes
r.GET("/", func(c *gin.Context) {
contextLogger(c).Infof("Main request successful")
c.String(http.StatusOK, "Hello World!")
})
r.GET("/favorites", func(c *gin.Context) {
// artificial sleep for delayTime
time.Sleep(time.Duration(delayTime) * time.Millisecond)
userID := c.Query("user_id")
contextLogger(c).Infof("Getting favorites for user %q", userID)
favorites, err := rdb.SMembers(c.Request.Context(), userID).Result()
if err != nil {
contextLogger(c).Error("Failed to get favorites for user %q", userID)
c.String(http.StatusInternalServerError, "Failed to get favorites")
return
}
contextLogger(c).Infof("User %q has favorites %q", userID, favorites)
c.JSON(http.StatusOK, gin.H{
"favorites": favorites,
})
})
// Start server
logger.Infof("App startup")
log.Fatal(http.ListenAndServe(":"+applicationPort, r))
logger.Infof("App stopped")
}
Step 2. Running the Docker image with environment variables
As specified in the OTEL documentation, we will use environment variables and pass in the configuration values that are found in your APM Agent’s configuration section.
Because Elastic accepts OTLP natively, we just need to provide the Endpoint and authentication where the OTEL Exporter needs to send the data, as well as some other environment variables.
Where to get these variables in Elastic Cloud and Kibana®
You can copy the endpoints and token from Kibana under the path /app/home#/tutorial/apm.
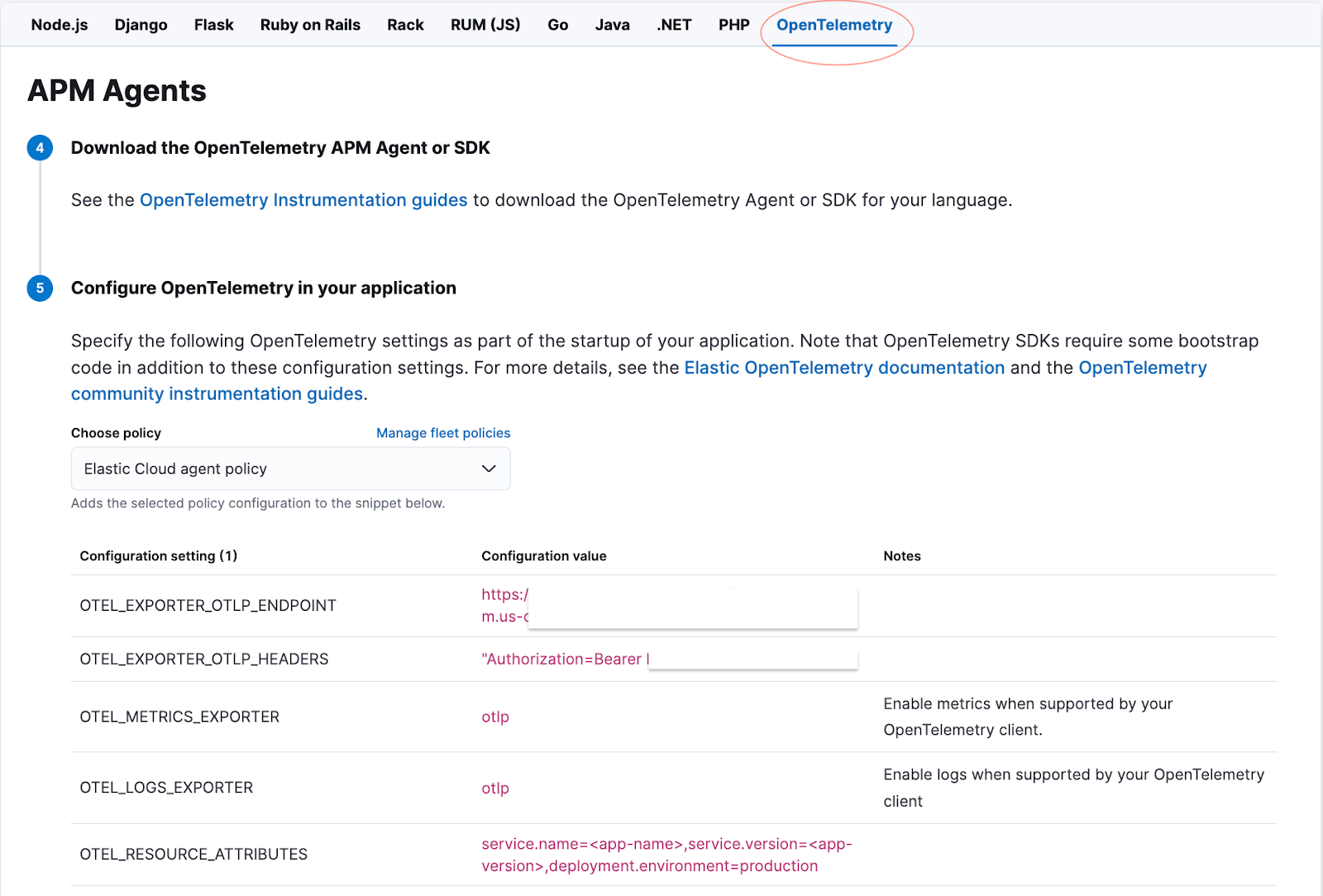
You will need to copy the OTEL_EXPORTER_OTLP_ENDPOINT as well as the OTEL_EXPORTER_OTLP_HEADERS.
Build the image
docker build -t go-otel-manual-image .
Run the image
docker run \
-e OTEL_EXPORTER_OTLP_ENDPOINT="<REPLACE WITH OTEL_EXPORTER_OTLP_ENDPOINT>" \
-e OTEL_EXPORTER_OTLP_HEADERS="Authorization=Bearer <REPLACE WITH TOKEN>" \
-e OTEL_RESOURCE_ATTRIBUTES="service.version=1.0,deployment.environment=production,service.name=go-favorite-otel-manual" \
-p 5000:5000 \
go-otel-manual-image
You can now issue a few requests in order to generate trace data. Note that these requests are expected to return an error, as this service relies on a connection to Redis that you don’t currently have running. As mentioned before, you can find a more complete example using Docker compose here.
curl localhost:500/favorites
# or alternatively issue a request every second
while true; do curl "localhost:5000/favorites"; sleep 1; done;
How do the traces show up in Elastic?
Now that the service is instrumented, you should see the following output in Elastic APM when looking at the transactions section of your Node.js service:
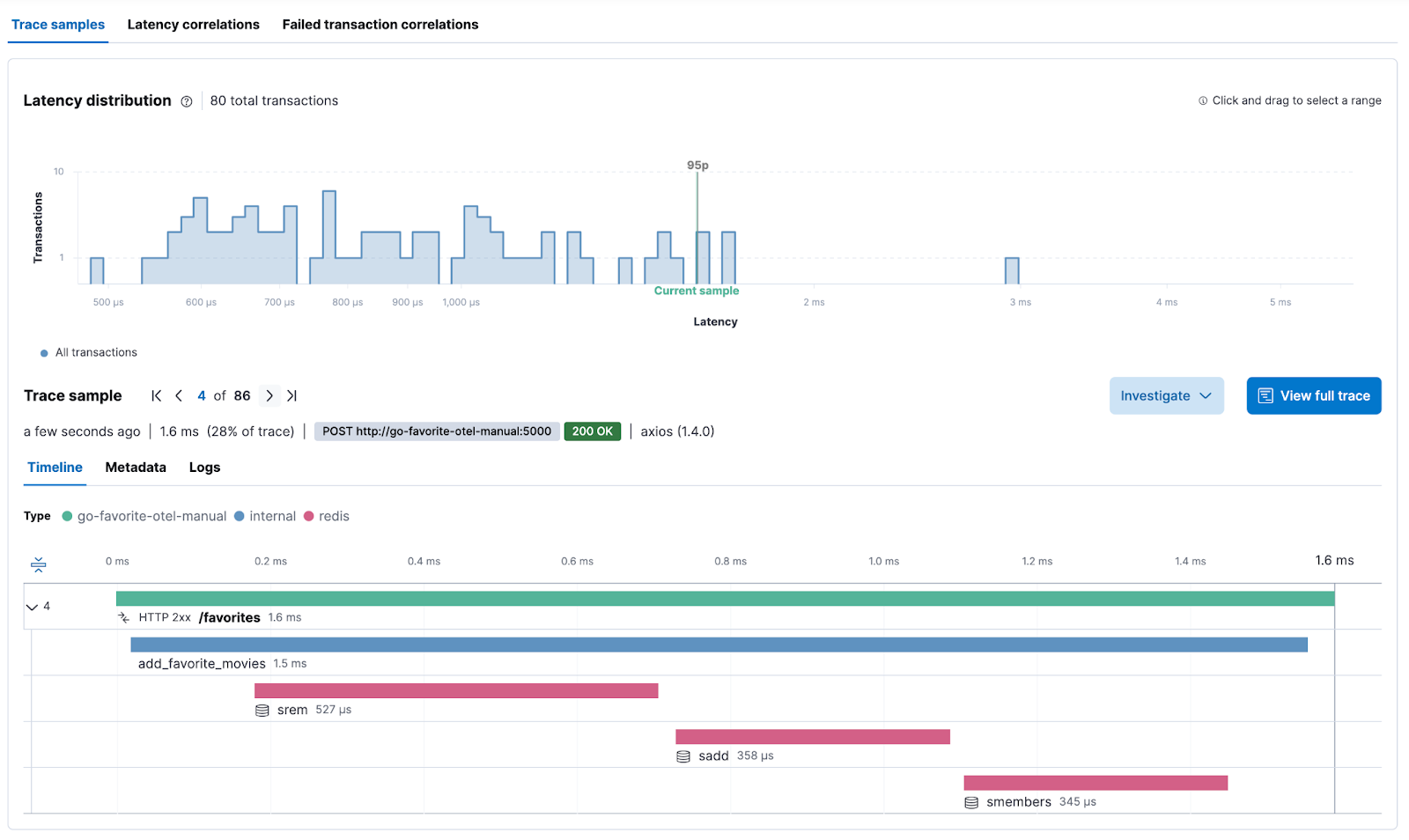
Conclusion
In this blog, we discussed the following:
- How to manually instrument Go with OpenTelemetry
- How to properly initialize OpenTelemetry and add a custom span
- How to easily set the OTLP ENDPOINT and OTLP HEADERS with Elastic without the need for a collector
Hopefully, this provides an easy-to-understand walk-through of instrumenting Go with OpenTelemetry and how easy it is to send traces into Elastic.
Additional resources for OpenTelemetry with Elastic:
Developer resources:
- Elastiflix application, a guide to instrument different languages with OpenTelemetry
- Python: Auto-instrumentation, Manual-instrumentation
- Java: Auto-instrumentation, Manual-instrumentation
- Node.js: Auto-instrumentation, Manual-instrumentation
- .NET: Auto-instrumentation, Manual-instrumentation
- Go: Manual-instrumentation
- Best practices for instrumenting OpenTelemetry
General configuration and use case resources:
- Independence with OpenTelemetry on Elastic
- Modern observability and security on Kubernetes with Elastic and OpenTelemetry
- 3 models for logging with OpenTelemetry and Elastic
- Adding free and open Elastic APM as part of your Elastic Observability deployment
- Capturing custom metrics through OpenTelemetry API in code with Elastic
- Future-proof your observability platform with OpenTelemetry and Elastic
- Elastic Observability: Built for open technologies like Kubernetes, OpenTelemetry, Prometheus, Istio, and more
Don’t have an Elastic Cloud account yet? Sign up for Elastic Cloud and try out the auto-instrumentation capabilities that I discussed above. I would be interested in getting your feedback about your experience in gaining visibility into your application stack with Elastic.
The release and timing of any features or functionality described in this post remain at Elastic's sole discretion. Any features or functionality not currently available may not be delivered on time or at all.