Monitoring Android applications with Elastic APM
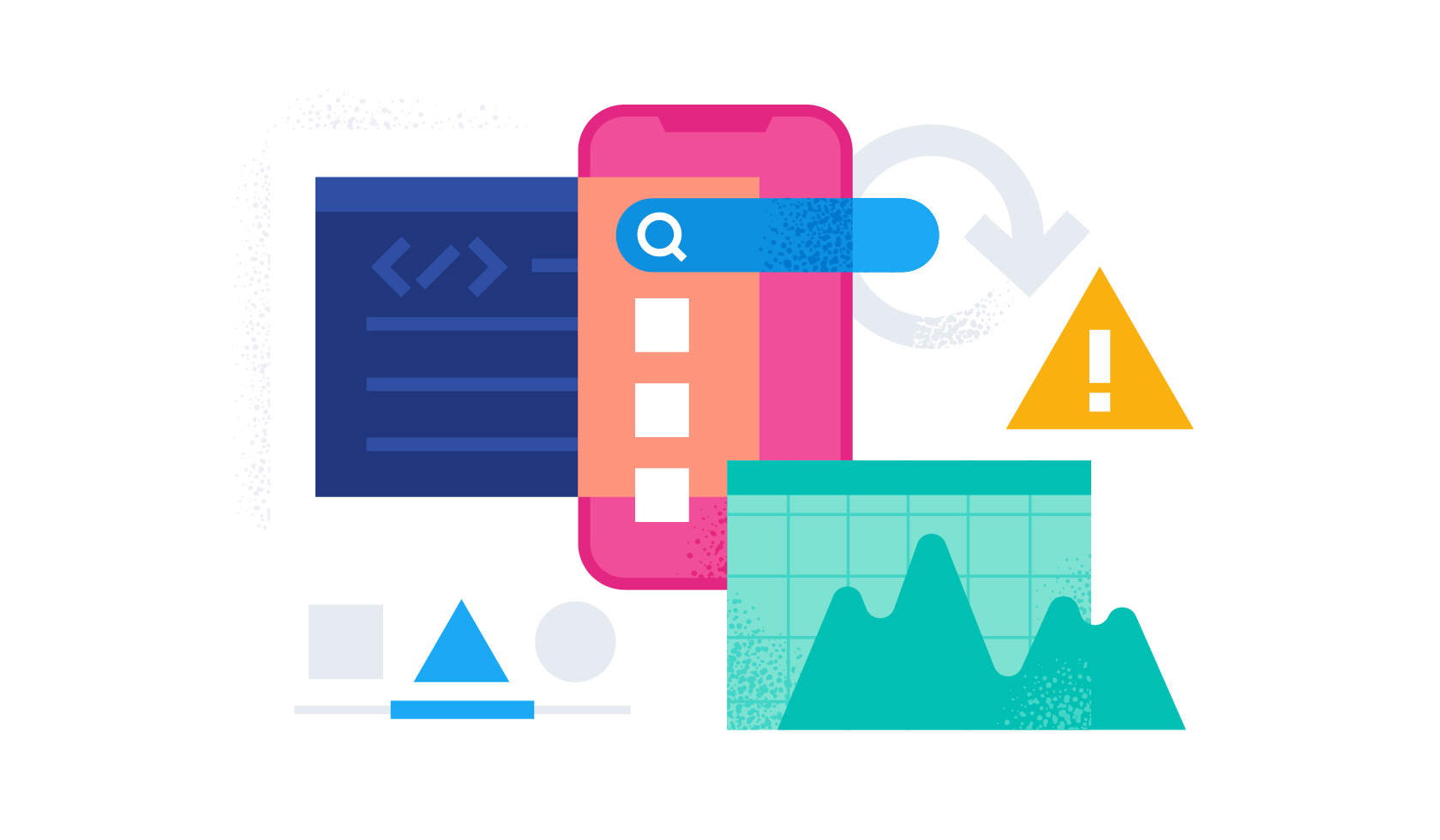
People are handling more and more matters on their smartphones through mobile apps both privately and professionally. With thousands or even millions of users, ensuring great monitor application performance and reliability is a key challenge for providers and operators of mobile apps and related backend services. Understanding the behavior of mobile apps, the occurrences and types of crashes, the root causes of slow response times, and the real user impact of backend issues is key to managing the performance of mobile apps and associated backend services.
Elastic has launched its application performance monitoring (APM) agent for Android applications, allowing developers to keep track of key aspects of their applications, from crashes and HTTP requests to screen rendering times and end-to-end distributed tracing. All of this helps troubleshoot issues and performance flaws with mobile applications, corresponding backend services, and their interaction. The Elastic APM Android Agent automatically instruments your application and its dependencies so that you can simply “plug-and-play” the agent into your application without having to worry about changing your codebase much.
The Elastic APM Android Agent has been developed from scratch on top of OpenTelemetry, an open standard and framework for observability. Developers will be able to take full advantage of its capabilities, as well as the support provided by a huge and active community. If you’re familiar with OpenTelemetry and your application is already instrumented with OpenTelemetry, then you can simply reuse it all when switching to the Elastic APM Android Agent. But no worries if that’s not the case — the agent is configured to handle common traceable scenarios automatically without having to deep dive into the specifics of the OpenTelemetry API.
[Related article: Adding free and open Elastic APM as part of your Elastic Observability deployment]
How it works
The Elastic APM Android Agent is a combination of an SDK plus a Gradle plugin. The SDK contains utilities that will let you initialize and configure the agent’s behavior, as well as prepare and initialize the OpenTelemetry SDK. You can use the SDK for programmatic configuration and initialization of the agent, in particular for advanced and special use cases.
In most cases, a programmatic configuration and initialization won’t be necessary. Instead, you can use the provided Gradle plugin to configure the agent and automatically instrument your app. The Gradle plugin uses Byte Buddy and the official Android Gradle plugin API under the hood to automatically inject instrumentation code into your app through compile-time transformation of your application’s and its dependencies’ classes.
Compiling your app with the Elastic Android APM Agent Gradle Plugin configured and enabled will make your Android app report tracing data, metrics, and different events and logs at runtime.
Using the Elastic APM Agent in an Android app
By means of a simple demo application, we’re going through the steps mentioned in the “Set up the Agent” guide to set up the Elastic Android APM Agent.
Prerequisites
For this example, you will need the following:
- An Elastic Stack with APM enabled (We recommend using Elastic’s Cloud offering. Try it for free.)
- Java 11+
- Android Studio
- Android Emulator, AVD device
You’ll also need a way to push the app’s signals into Elastic. Therefore, you will need Elastic APM’s secret token that you’ll configure into our sample app later.
Test project for our example
To showcase an end-to-end scenario including distributed tracing, in this example, we’ll instrument a simple weather application that comprises two Android UI fragments and a simple local backend service based on Spring Boot.
The first fragment will have a dropdown list with some city names and also a button that takes you to the second one, where you’ll see the selected city’s current temperature. If you pick a non-European city on the first screen, you’ll get an error from the (local) backend when you head to the second screen. This is to demonstrate how network and backend errors are captured and correlated in Elastic APM.
Applying the Elastic APM Agent plugin
In the following, we will explain all the steps required to set up the Elastic APM Android Agent from scratch for an Android application. In case you want to skip these instructions and see the agent in action right away, use the main branch of that repo and apply only Step (3.b) before continuing with the next Section (“Setting up the local backend service”).
1. Clone the sample app repo and open it in Android Studio.
2. Switch to the uninstrumented repo branch to start from a blank, uninstrumented Android application. You can run this command to switch to the uninstrumented branch:
git checkout uninstrumented
3. Follow the Elastic APM Android Agent’s setup guide:
Add the co.elastic.apm.android plugin to the app/build.gradle file (please make sure to use the latest version available of the plugin, which you can find here).
Configure the agent’s connection to the Elastic APM backend by providing the ‘serverUrl’ and ‘secretToken’ in the ‘elasticAPM’ section of the app/build.gradle file.
// Android app's build.gradle file
plugins {
//...
id "co.elastic.apm.android" version "[latest_version]"
}
//...
elasticApm {
// Minimal configuration
serverUrl = "https://your.elastic.apm.endpoint"
// Optional
serviceName = "weather-sample-app"
serviceVersion = "0.0.1"
secretToken = "your Elastic APM secret token"
}
4. The only actual code change required is a one-liner to initialize the Elastic APM Android Agent in the Application.onCreate method. The application class for this sample app is located at app/src/main/java/co/elastic/apm/android/sample/MyApp.kt.
package co.elastic.apm.android.sample
import android.app.Application
import co.elastic.apm.android.sdk.ElasticApmAgent
class MyApp : Application() {
override fun onCreate() {
super.onCreate()
ElasticApmAgent.initialize(this)
}
}
Bear in mind that for this example, we’re not changing the agent’s default configuration — if you want more information about how to do so, take a look at the agent’s runtime configuration guide.
Before launching our Android Weather App, we need to configure and start the local weather-backend service as described in the next section.
Setting up the local backend service
One of the key features the agent provides is distributed tracing, which allows you to see the full end-to-end story of an HTTP transaction, starting from our mobile app and traversing instrumented backend services used by the app. Elastic APM will show you the full picture as one distributed trace, which comes in very handy for troubleshooting issues, especially the ones related to high latency and backend errors.
As part of our sample app, we’re going to launch a simple local backend service that will handle our app’s HTTP requests. The backend service is instrumented with the Elastic APM Java agent to collect and send its own APM data over to Elastic APM, allowing it to correlate the mobile interactions with the processing of the backend requests.
In order to configure the local server, we need to set our Elastic APM endpoint and secret token (the same used for our Android app in the previous step) into the backend/src/main/resources/elasticapm.properties file:
service_name=weather-backend
application_packages=co.elastic.apm.android.sample
server_url=YOUR_ELASTIC_APM_URL
secret_token=YOUR_ELASTIC_APM_SECRET_TOKEN
Launching the demo
Our sample app will get automatic instrumentation for the agent’s currently supported frameworks, which means that we’ll get to see screen rendering spans as well as OkHttp requests out of the box. For frameworks not currently supported, you could apply manual instrumentation to enrich your APM data (see “Manual Instrumentation” below).
We are ready to launch the demo. (The demo is meant to be executed on a local environment using an emulator for Android.) Therefore, we need to:
- Launch the backend service using this command in a terminal located in the root directory of our sample project: ./gradlew bootRun (or gradlew.bat bootRun if you’re on Windows). Alternatively, you can start the backend service from Android Studio.
- Launch the weather sample app in an Android emulator (from Android Studio).
Once everything is running, we need to navigate around in the app to generate some load that we would like to observe in Elastic APM. So, select a city, click Next and repeat it multiple times. Please, also make sure to select New York at least once. You will see that the weather forecast won’t work for New York as the city. Below, we will use Elastic APM to find out what’s going wrong when selecting New York.
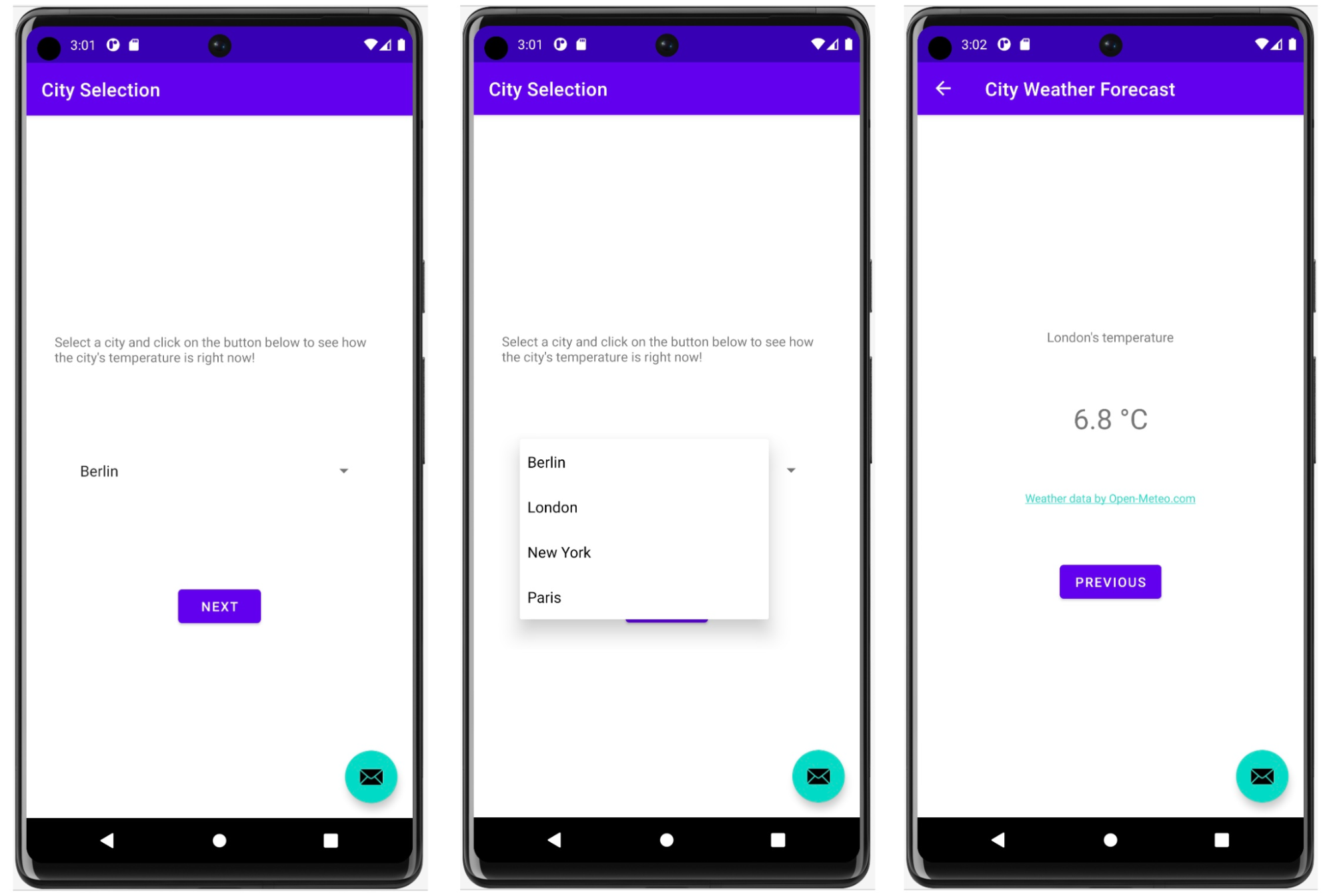
First glance at the APM results
Let’s open Kibana and navigate to the Observability solution.
Under the Services navigation item, you should see a list of two services: our Android app weather-sample-app and the corresponding backend service weather-backend. Click on the Service map tab to see a visualization of the dependencies between those services and any external services.
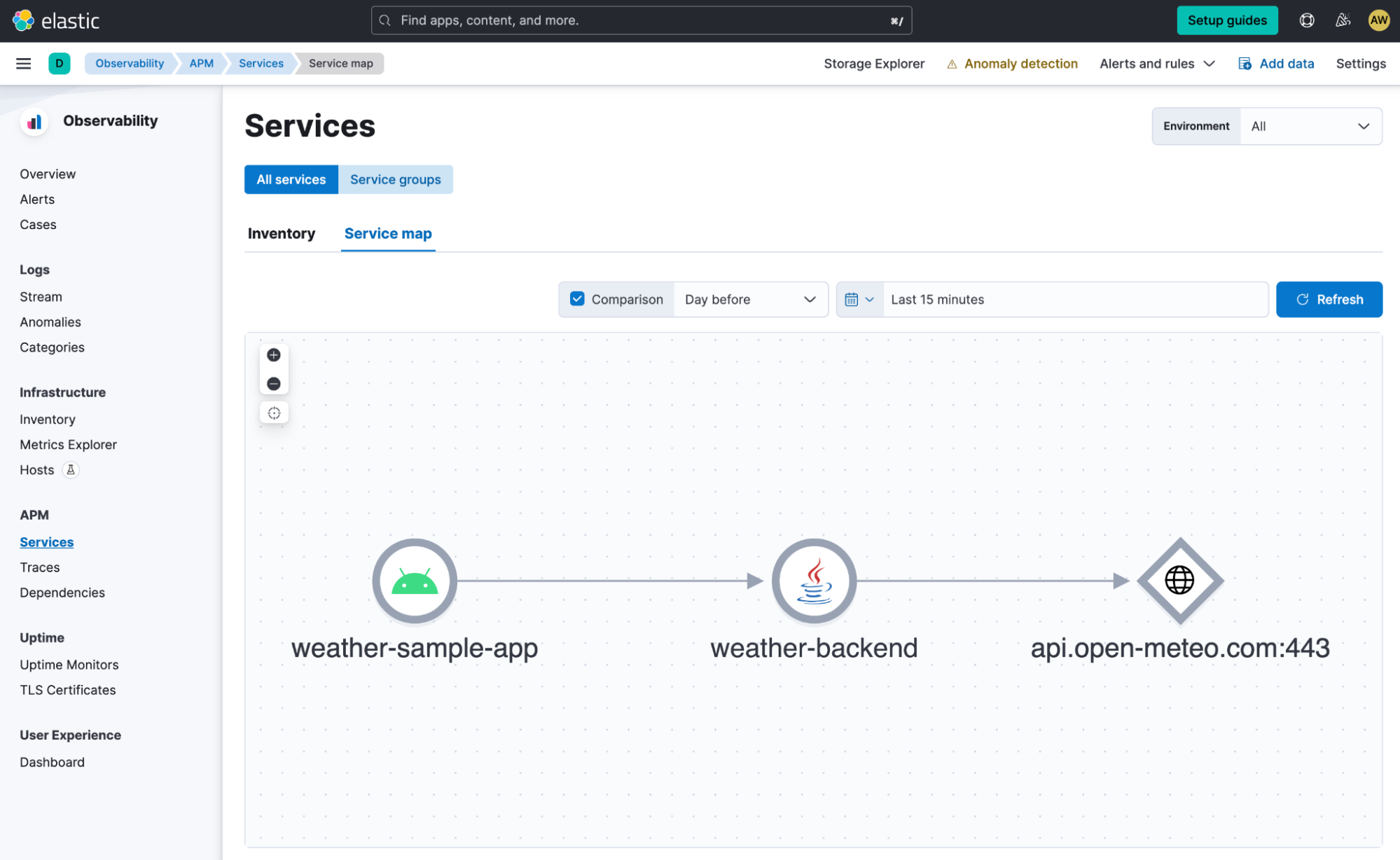
Click on the weather-sample-app to dive into the dashboard for the Android app. The service view for mobile applications is in technical preview at the publishing of this blog post, but you can already see insightful information about the app on that screen. You see information like the amount of active sessions in the selected time frame, number of HTTP requests emitted by the weather-sample-app, geographical distribution of the requests as well as breakdowns on device models, OS versions, network connection types, and app versions. (Information on crashes and app load times are under development.)
For the purpose of demonstration, we kept this demo simple, so the data is less diversified and also rather limited. However, this kind of data is particularly useful when you are monitoring a mobile app with higher usage numbers and higher diversification on device models, OS versions, etc. Troubleshooting problems and performance issues becomes way easier when you can use these properties to filter and group your APM data. You can use the quick filters at the top to do so and see how the metrics adopt depending on your selection.
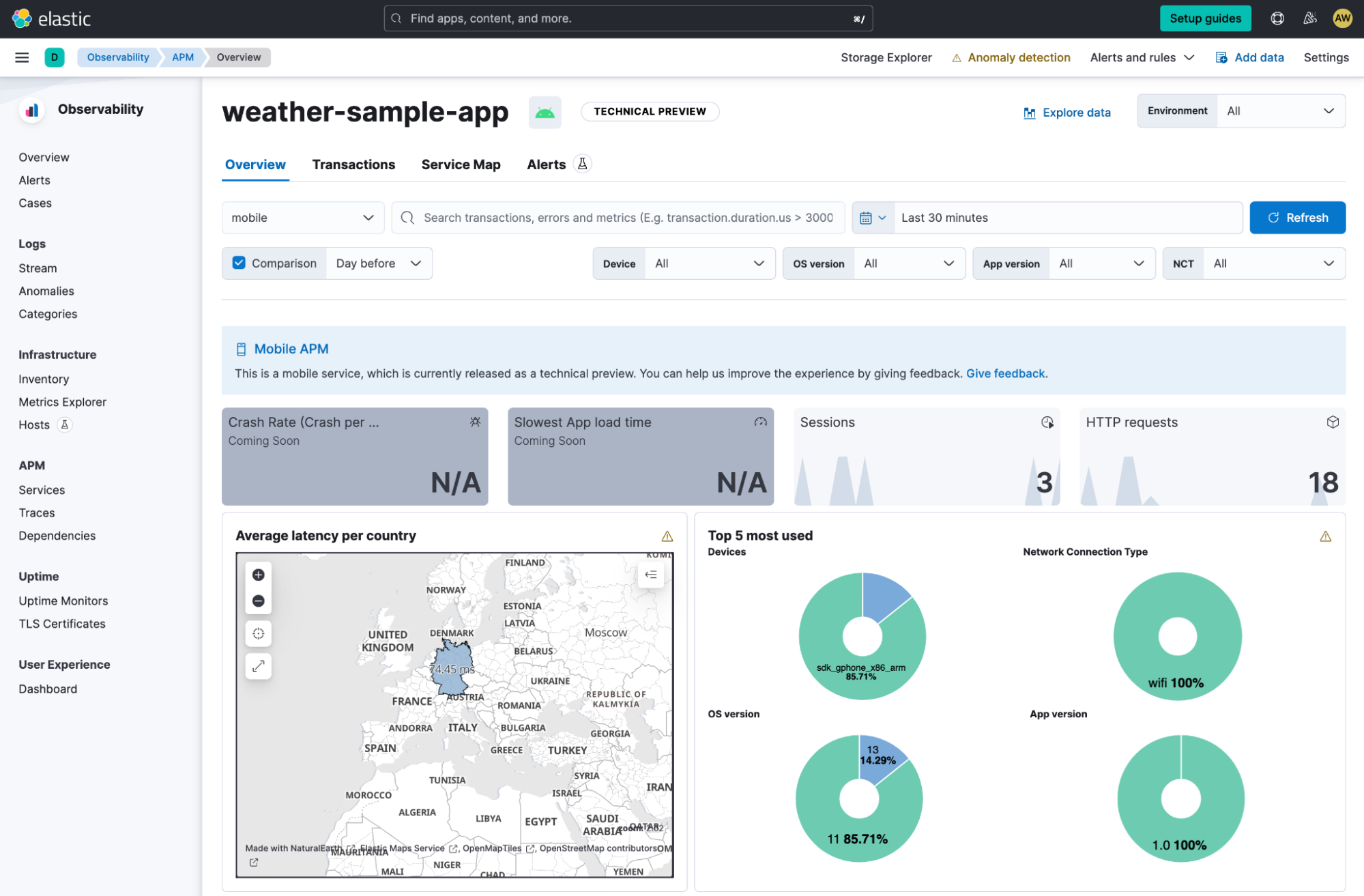
Now, let’s see how individual user interactions are processed, including downstream calls into the backend service. Under the Transactions tab (at the top), we see the different end-to-end transaction groups, including the two transactions for the FirstFragment and the SecondFragment.
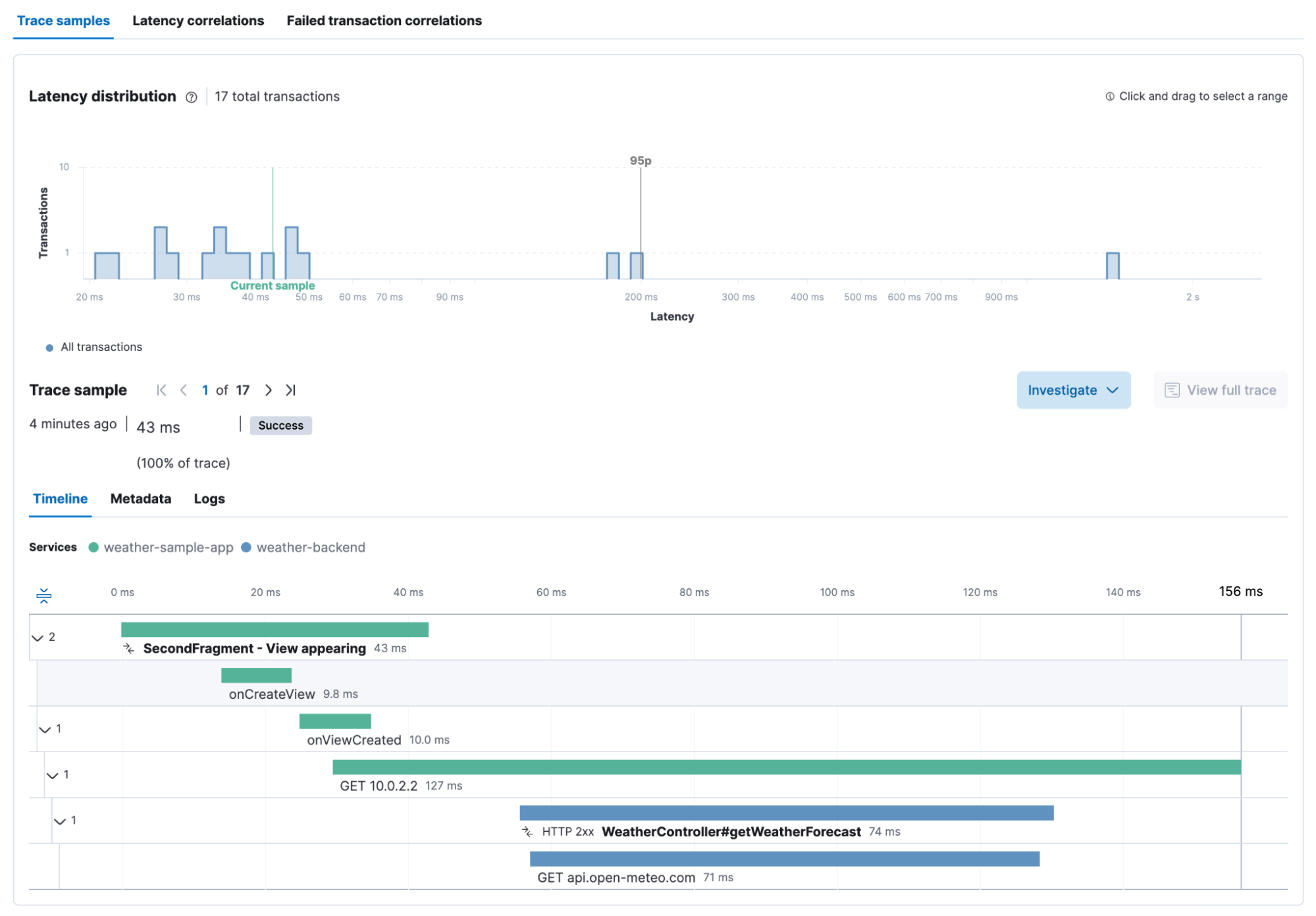
Let’s deep dive into the SecondFragment - View appearing transaction, to see the metrics (e.g., latency, throughput) for this transaction group and also the invocation waterfall view for the individual user interactions. As we can see in the following screenshot, after view creation, the fragment performs an HTTP GET request to 10.0.2.2, which takes ~130 milliseconds. In the same waterfall, we see that the HTTP call is processed by the weather-backend service, which itself conducts an HTTP call to api.open-meteo.com.
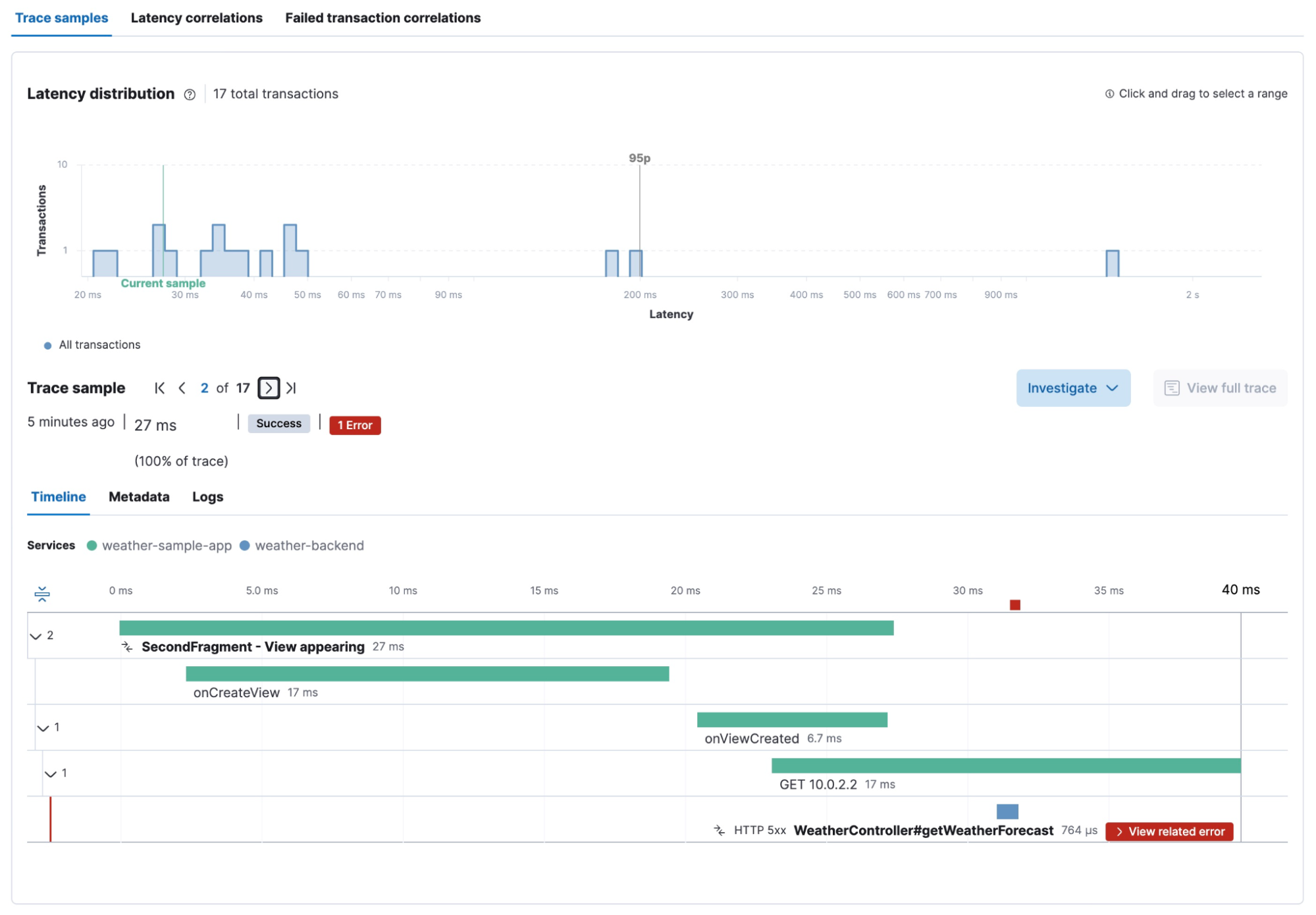
Now, when looking at the waterfall view for a request where New York was selected as the city, we see an error happening on the backend service that explains why the forecast didn’t work for New York. By clicking on the red View related error badge, you will get details on the error and the actual root cause of the problem.
The exception message on the weather-backend states that “This service can only retrieve geo locations for European cities!” That’s the problem with selecting New York as the city.
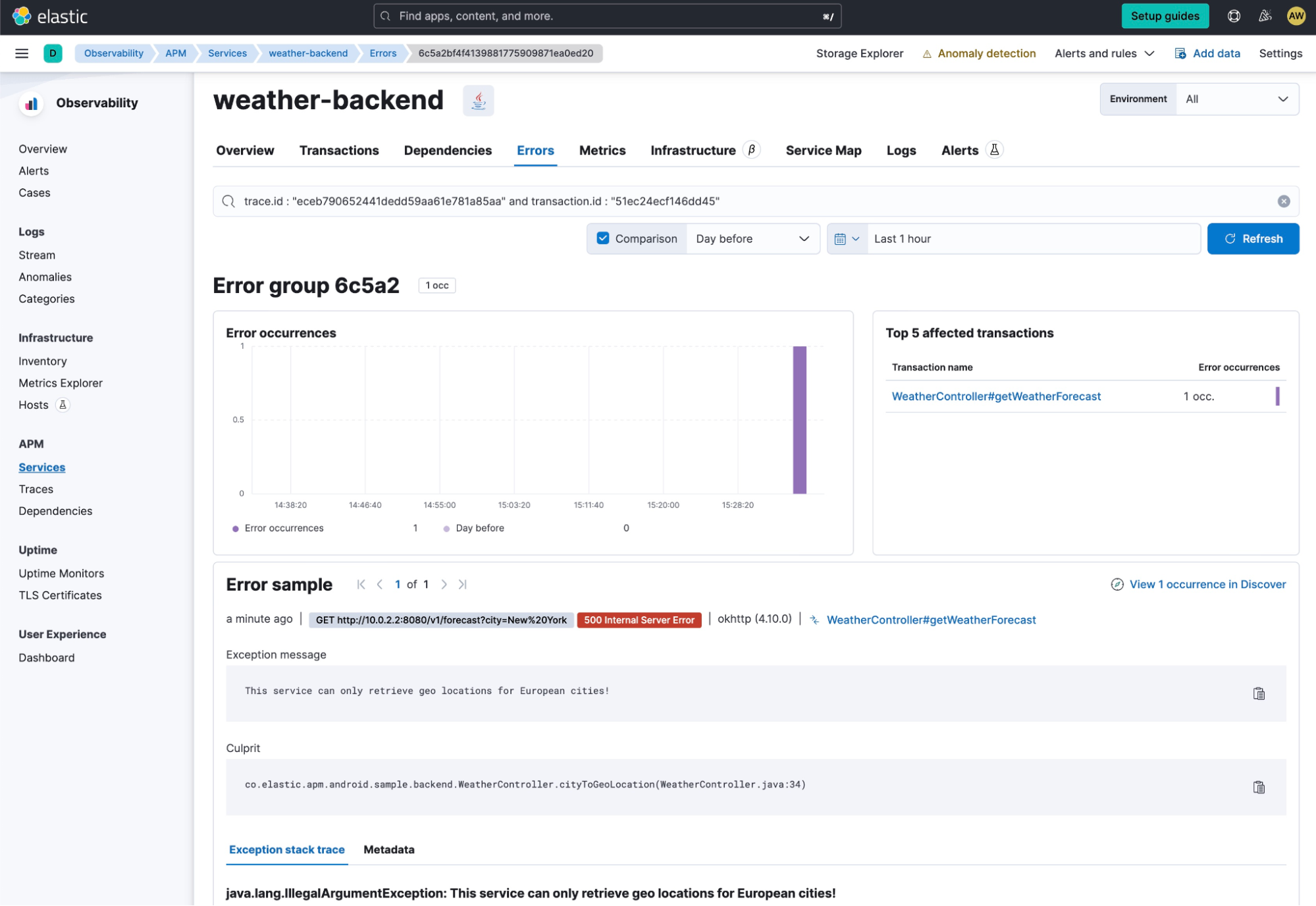
Manual instrumentation
As previously mentioned, the Elastic APM Android Agent does a bunch of automatic instrumentation on your behalf for the supported frameworks; however, in some cases, you might want to get extra instrumentation depending on your app’s use cases. For those cases, you’ve gotten covered by the OpenTelemetry API, which is what the Elastic APM Android Agent is based on. The OpenTelemetry Java SDK contains tools to create custom spans, metrics, and logs, and since it’s the base of the Elastic APM Android Agent, it’s available for you to use without having to add any extra dependencies into your project and without having to configure anything to connect your custom signals to your own Elastic environment either, as the agent does that for you.
The way to start would be by getting OpenTelemetry’s instance like so:
OpenTelemetry openTelemetry = GlobalOpenTelemetry.get();
And then you can follow the instructions from the OpenTelemetry Java documentation in order to create your custom signals. See the following example for the creation of a custom span:
OpenTelemetry openTelemetry = GlobalOpenTelemetry.get();
Tracer tracer = openTelemetry.getTracer("instrumentation-library-name", "1.0.0");
Span span = tracer.spanBuilder("my span").startSpan();
// Make the span the current span
try (Scope ss = span.makeCurrent()) {
// In this scope, the span is the current/active span
} finally {
span.end();
}
Conclusion
In this blog post, we demonstrated how you can use the Elastic APM Android Agent to achieve end-to-end observability into your Android-based mobile applications. Setting up the agent is a matter of a few minutes and the provided insights allow you to analyze your app’s performance and its dependencies on backend services. With the Elastic APM Android Agent in place, you can leverage Elastic’s rich APM feature as well as the various possibilities to customize your analysis workflows through custom instrumentation and custom dashboards.
Are you curious? Then try it yourself. Sign up for a free trial on the Elastic Cloud, enrich your Android app with the Elastic APM Android agent as described in this blog, and explore the data in Elastic’s Observability solution.