Bringing Elastic to robotics developers: Integrating with ROS
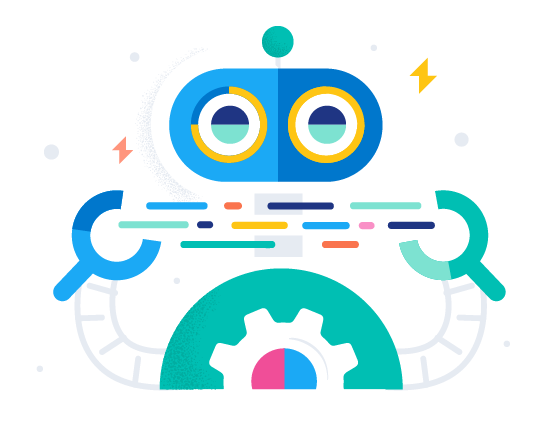
The robotics industry has experienced significant growth in recent years, with the global robotics market size expected to reach US$230 billion by 2025. This growth is largely driven by the increasing adoption of automation and robotics in various industries, including manufacturing, healthcare, logistics, and more. The integration of automation and robotics with Industry 4.0 technologies has brought new challenges, including the management and analysis of large amounts of data generated by connected devices and sensors, which is estimated to generate around 4.4 zettabytes of data per year.
The Robot Operating System (ROS) is an open-source framework that provides a standardized way of developing robotic applications. ROS has become an essential component of the robotics industry, as it simplifies the process of building and deploying robotic systems. With ROS, developers can easily integrate sensors, hardware, and other components to build complex robotic systems. But how can developers integrate ROS data inside Elastic?
Putting ROS data to work in Elastic
The increasing amount of data generated by the Industrial Internet of Things (IIoT) presents a major challenge for the robotics industry. The sheer volume of data generated by connected devices and sensors can be overwhelming, making it difficult to manage, process, and analyze. In order to effectively harness the insights provided by this data, organizations need a platform that is robust and sufficiently scalable to handle large amounts of data in real time.
Some companies are using Elastic to help with these issues. MM Karton and REHAU use Elastic to optimize their production processes and improve the behavior of their robots, respectively. TRACTO minimizes downtime and Mean Time to Repair (MTTR) to enable customers to complete projects faster, while Clearpath Robotics gains insights into the performance of its robots to improve operations. Many other companies need to power their IIoT platform, optimizing their operations and providing valuable insights to customers.
ROS structure
The Robot Operating System (ROS) is based on a distributed architecture, which consists of a set of nodes that communicate with each other using a publish-subscribe messaging system. Each node is responsible for performing a specific task, such as processing sensor data, controlling a robot's motion, or displaying visual feedback.
Nodes in ROS communicate with each other by publishing messages on topics. Topics are named channels that allow nodes to send and receive data in a standardized format. For example, a node that processes sensor data may publish sensor readings on a topic, while a node that controls a robot's motion may subscribe to that topic to receive the sensor data and use it to adjust the robot's movement.
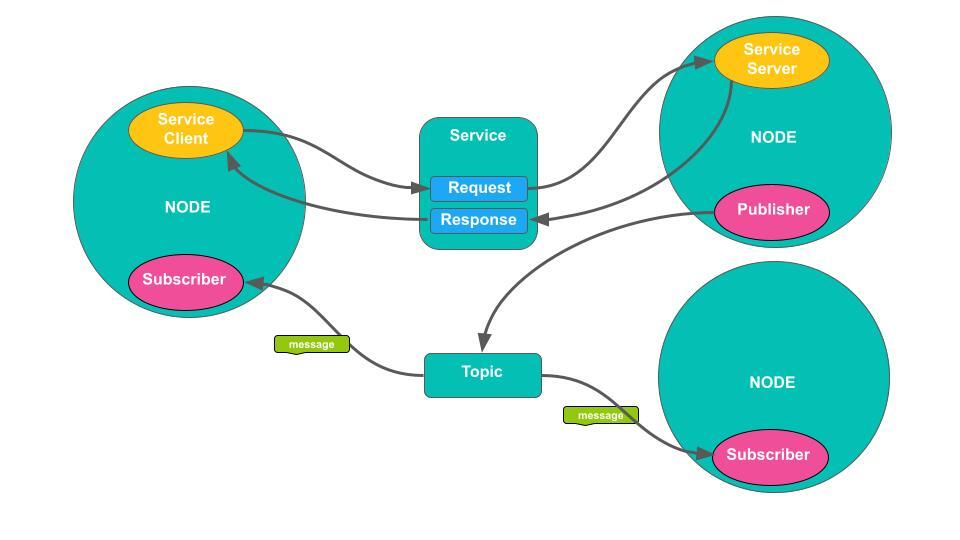
Prerequisites
To prepare the environment for ROS/Elastic, you’ll need:
1. A machine with ROS installed. In our example:
- Ubuntu 22.04
- ROS 2 Humble (For more information, refer to the official documentation: https://docs.ros.org/en/humble/index.html.)
- Python 3.x
2. A machine to install Elastic Filebeat (it could be the same machine or a separate one). In my case, I used:
- A MacBook Pro
- Filebeat
- A MQTT broker
3. A registered account on Elastic Cloud, which I will use to store data.
4. A robot. But don't worry, we will use a simulator of those cleaning robots. The TurtleBot3 running in Gazebo is well-known among ROS developers.
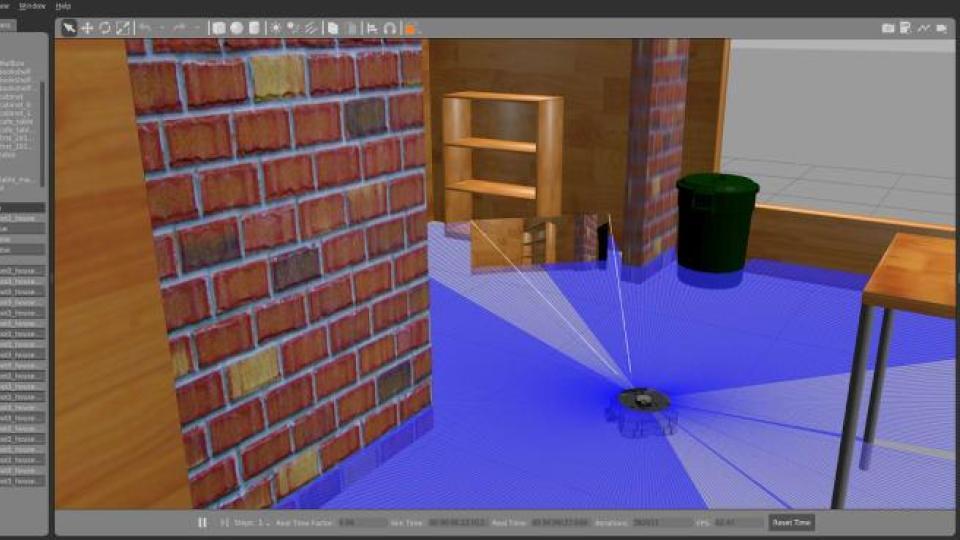
Ingesting sensor data: How to use ROS with Elastic
In our example, retrieving data from sensors in real time or storing a log of data to be analyzed is one of the big challenges for robotics developers. Since this data is normally on edge computers, a centralized and robust platform to solve this problem is needed. So, we are proposing the following architecture:
ROS architecture: Nodes and topics
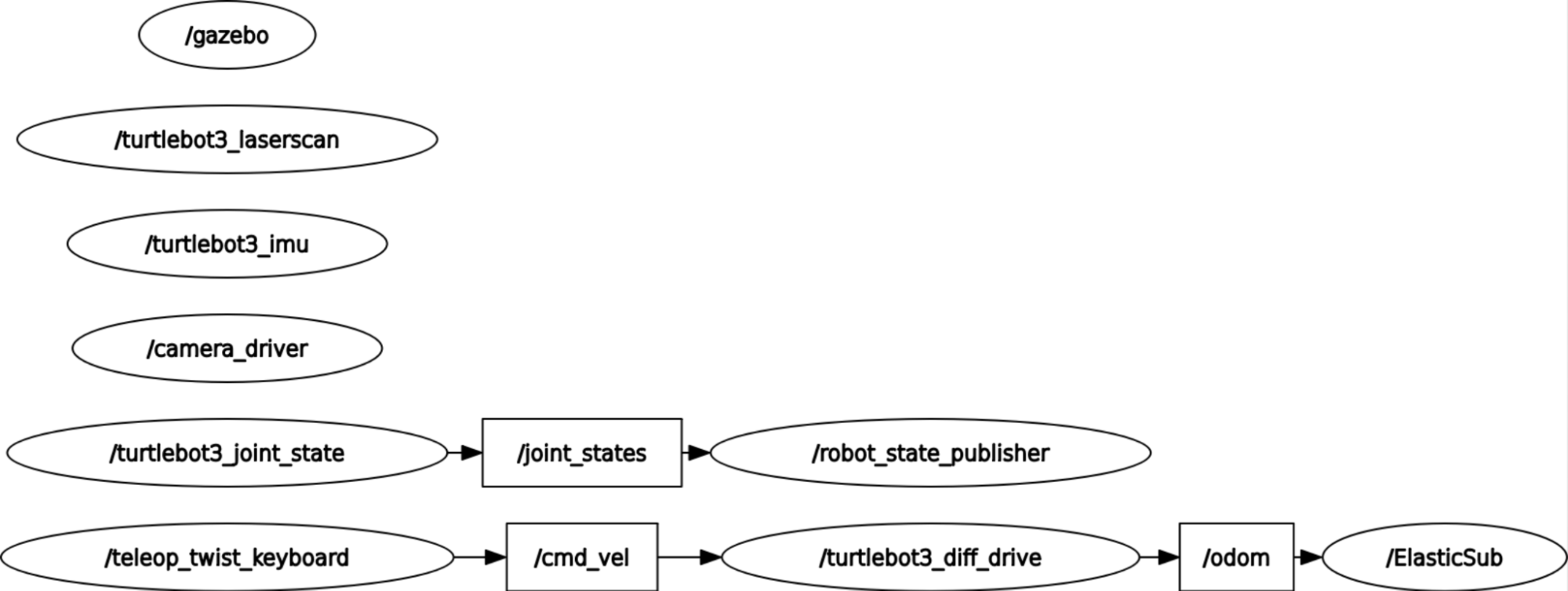
For ROS, we will create a node called ElasticSub. This node will perform the job of listening to a sensor topic /odom and publish it on the MQTT broker. From the Elastic side, we will use Filebeat to ingest the data from MQTT to Elasticsearch. The main architecture is as follows:
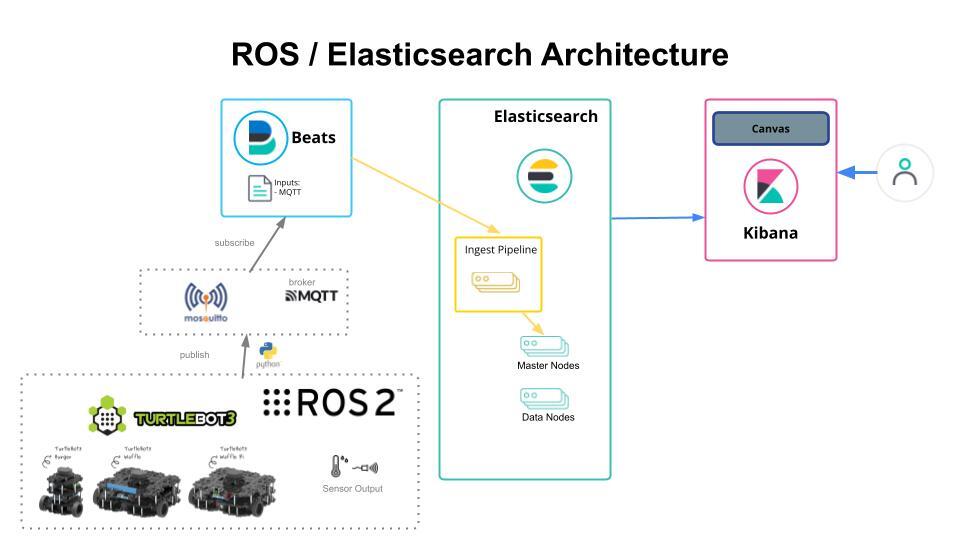
Implementation
To implement the architecture mentioned above, let's follow these steps:
Step 1. Code for ROS NODE
To assist with the initial installation, the main code can be downloaded from GitHub as shown in the script below:
# open a terminal - build elastic node
cd ~
git clone https://github.com/salgado/blog.elastic.ros.git
cd blog.elastic.ros
cd ros2_ws
#build ros
colcon build --symlink-install
source install/setup.bash
The main code, node_elastic.py, is located in the folder
ros2_ws/src/pkg_elastic/pkg_elastic. In it, we can highlight:
- Subscription to the /odom topic
class ElasticSub(Node):
def pub_mqtt(self, msg_json):
self._elastic_mqtt.publish(msg_json)
def __init__(self):
super().__init__('ElasticSub')
self.subscription = self.create_subscription(
Odometry,
'/odom',
self.listener_callback,
10)
self.subscription # prevent unused variable warning
broker = 'ec2-3-82-196-231.compute-1.amazonaws.com'
port = 1883
topic = "robot/robot01"
self._elastic_mqtt = ElasticMqtt(broker, port, topic)
self._elastic_mqtt.run()
MQTT is a lightweight messaging protocol designed to send messages between devices with limited processing and bandwidth resources. It is commonly used in IoT (Internet of Things) applications, where there are large numbers of connected devices that need to exchange information in real time.
The MQTT broker can be hosted in the cloud or installed on a local server, depending on the user's needs. It typically offers features such as authentication, security, and access control, allowing users to manage and monitor MQTT connections in a centralized way.
There are some MQTT brokers ready to be used for testing, such as "test.mosquitto.org" always using port 1883 as the default access via TCP, but if you want more security, there are other options for ports with authentication and encryption.
For our experiment, I created and installed an EC2 machine on AWS and started Mosquitto, making it available at the following address.
broker = 'ec2-3-82-196-231.compute-1.amazonaws.com'
Therefore, using the Eclipse Paho MQTT python library, it is possible to publish and subscribe to the topic using the broker address.
- Callback routine to publish on MQTT
def listener_callback(self, msg):
self.get_logger().info("Odom:")
# Extract relevant values from message
x = msg.pose.pose.position.x
y = msg.pose.pose.position.y
z = msg.pose.pose.position.z
qx = msg.pose.pose.orientation.x
qy = msg.pose.pose.orientation.y
qz = msg.pose.pose.orientation.z
qw = msg.pose.pose.orientation.w
# Print extracted values
self.get_logger().info(f"Position: ({x:.2f}, {y:.2f}, {z:.2f})")
self.get_logger().info(f"Orientation: ({qx:.2f}, {qy:.2f}, {qz:.2f}, {qw:.2f})")
# Create a dictionary with the extracted values
data = {"odom": {
"position": {"x": round(x, 2), "y": round(y, 2), "z": round(z, 2)},
"orientation": {"x": round(qx, 2), "y": round(qy, 2), "z": round(qz, 2), "w": round(qw, 2)},
}}
# Convert the dictionary to a JSON object
json_data = json.dumps(data)
# Publish the JSON data to the MQTT topic
self.pub_mqtt(json_data)
- Publication on MQTT:
class ElasticMqtt:
def __init__(self, broker, port, topic):
self.broker = broker
self.port = port
self.topic = topic
self.client_id = f'python-mqtt-{random.randint(0, 1000)}'
self.client = self.connect_mqtt()
def on_connect(self, client, userdata, flags, rc):
if rc == 0:
print("Connected to MQTT Broker!")
else:
print("Failed to connect, return code %d\n", rc)
def connect_mqtt(self):
client = mqtt_client.Client(self.client_id)
client.on_connect = self.on_connect
client.connect(self.broker, self.port)
return client
def publish(self, msg):
result = self.client.publish(self.topic, msg)
status = result[0]
if status == 0:
print(f"Send `{msg}` to topic `{self.topic}`")
else:
print(f"Failed to send message to topic {self.topic}")
Step 2. Code for integration
On the Elastic platform side, after installing Filebeat on your machine following the official documentation instructions, we need to configure the filebeat.yml and create a data ingestion pipeline within the platform.
Configuring the filebeat.yml
filebeat.inputs:
- type: mqtt
hosts: ["tcp://ec2-3-82-196-231.compute-1.amazonaws.com:1883"]
topics: ["robot/robot01"]
tags: ["blog"]
fields:
fleet_id: "robot"
fields_under_root: true
Here we tell the Filebeat to use the MQTT protocol at the address "tcp://ec2-3-82-196-231.compute-1.amazonaws.com:1883" to listen to the topic "robot/robot01" and start data ingestion.
# ----------------------- Elasticsearch Output --------------------------
cloud.id: "your_id"
cloud.auth: "your_user:your_password"
output.elasticsearch:
index: "robot-data-%{+yyyy.MM.dd}"
pipeline: mqtt-ros
setup.template.name: "robot-data"
setup.template.pattern: "robot-data-*"
setup.ilm.overwrite: true
The final configuration of the Filebeat is to specify the authentication and login credentials (in this case, Elastic Cloud), the index name, and the name of the pipeline that will process the data upon arrival.
Creating the data ingestion pipeline
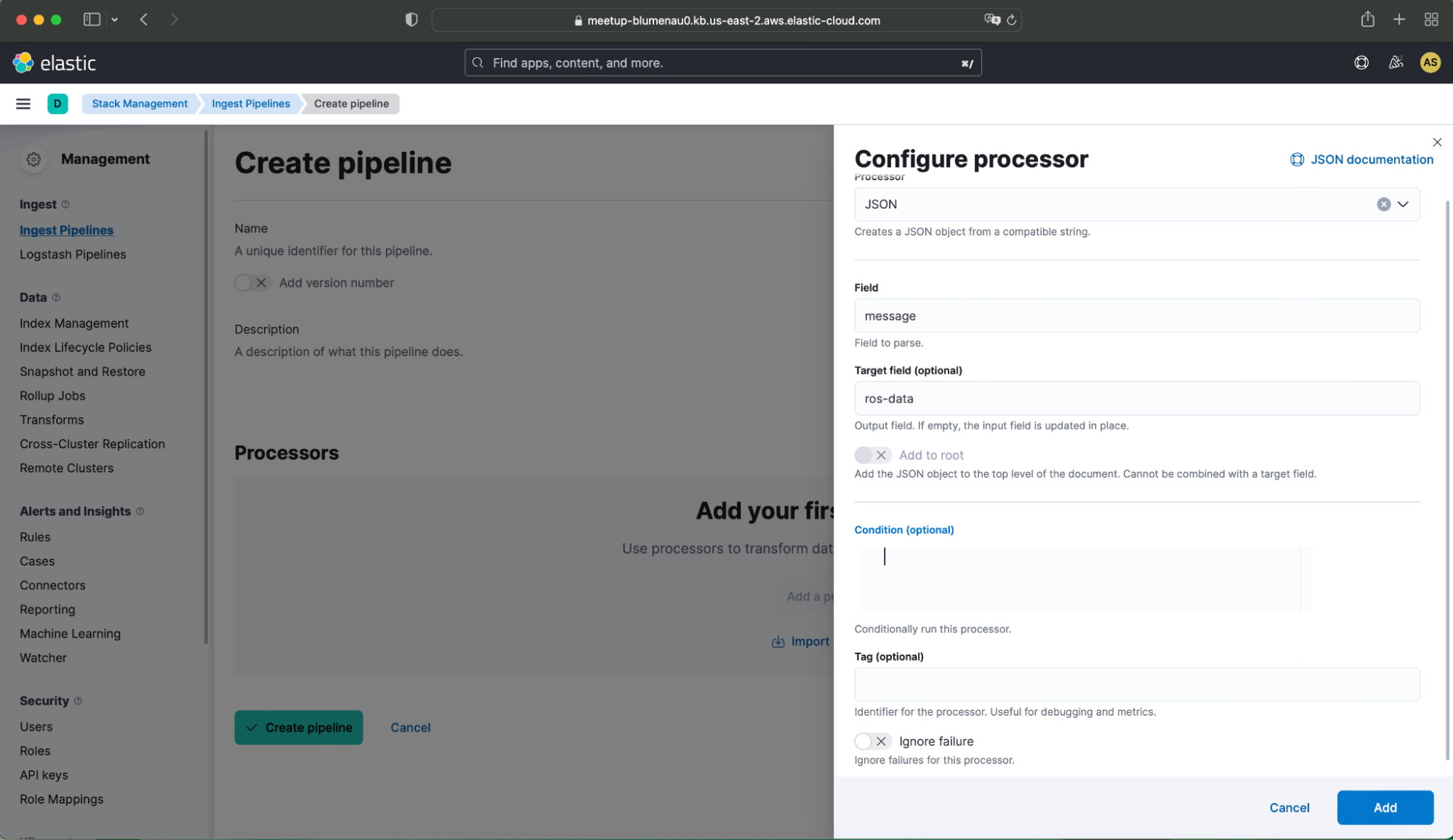
Here, we create a data ingestion pipeline called "mqtt-ros" where we set it up to receive a JSON message and transform it into attributes of the index in Elasticsearch.
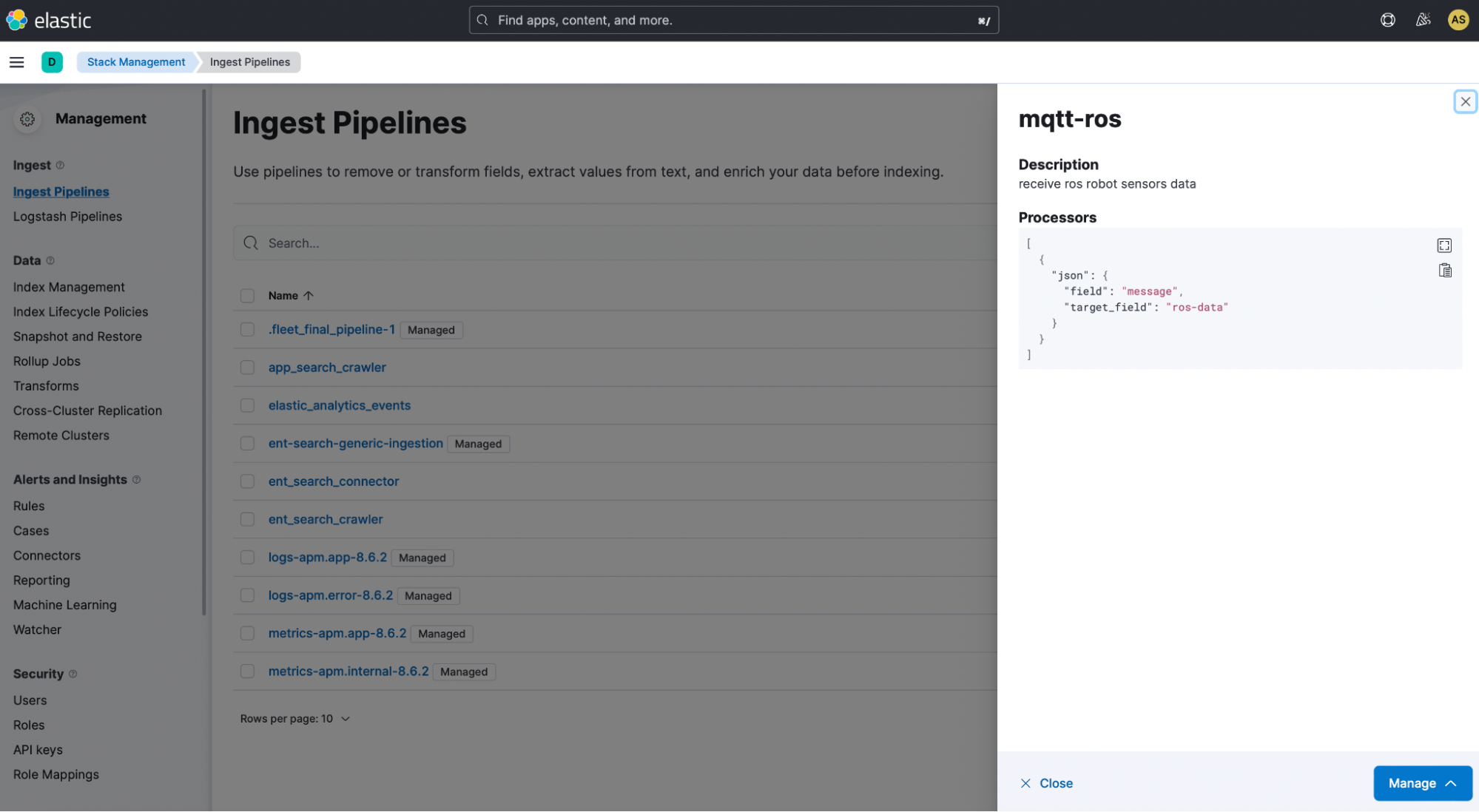
Step 3. Visualize data in Elasticsearch like magic
Now it's time to make the magic happen!
On the machine where the Filebeat is installed, open the terminal and type the following commands:
# test filebeat
./filebeat test config
./filebeat test output
./filebeat setup
# start filebeat
./filebeat -e
On the machine where ROS is installed, start three terminal screens for better organization and in each terminal, initialize the robot simulation with the following commands:
# open terminal 1 - run turtlebot3 on gazebo
ros2 launch turtlebot3_gazebo turtebot3_world.launch.py
# open terminal 2 - control teleop
ros2 run teleop_twist_keyboard teleop_twist_keyboard
# open terminal 3
ros2 run pkg_elastic node_elastic
Done! At this moment, our Turtlebot3 cleaning robot is walking around the room and transmitting its position in real time to the Filebeat through the MQTT protocol. This, in turn, performs a small transformation on the data and sends it to the index in Elasticsearch.
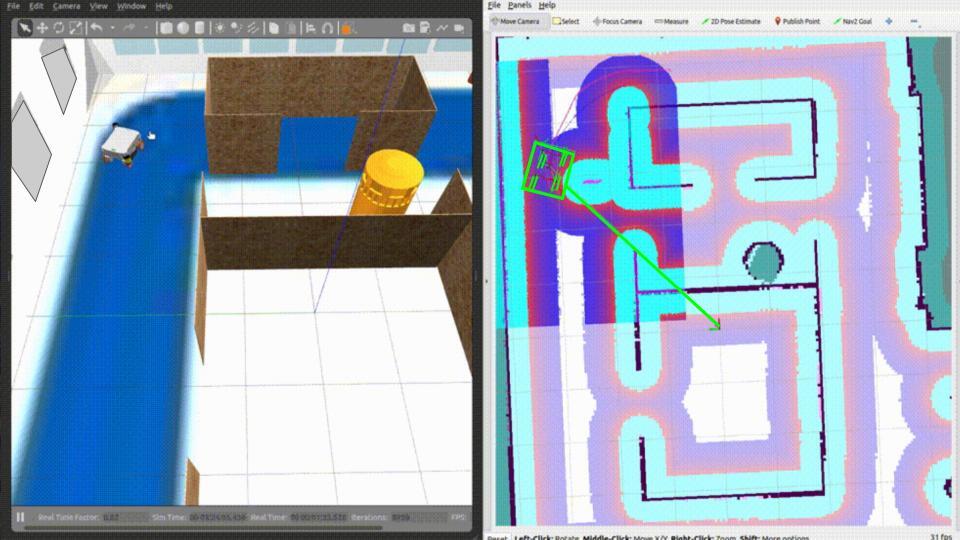
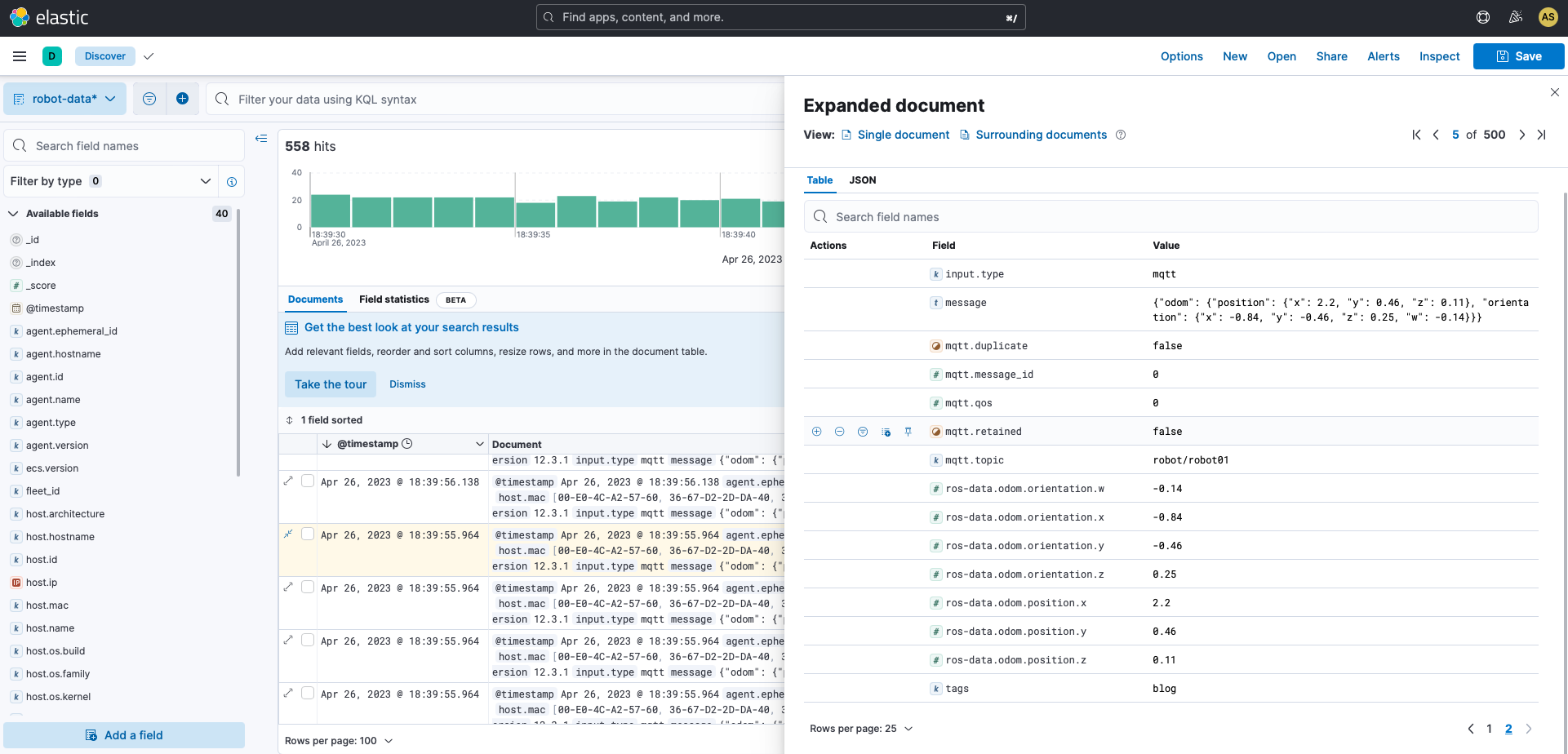
Conclusion
In conclusion, the integration of Elastic with ROS provides a scalable and robust solution for managing and analyzing the large amounts of data generated by connected devices and sensors in the robotics industry. With the proposed architecture, developers can easily ingest sensor data in real time or store it for later analysis, providing valuable insights to improve the behavior and performance of robotic systems.
By creating a ROS node that listens to a sensor topic and publishes it on the MQTT broker, and configuring Filebeat to ingest data from MQTT to Elasticsearch, developers can easily visualize data in Elasticsearch and gain insights into the behavior of their robots. With the increasing adoption of automation and robotics in various industries, the integration of Elastic with ROS provides a powerful tool for developers to optimize their operations and improve the performance of their robotic systems.
As the robotics industry grows and evolves, the integration of Elastic with ROS will continue to play a crucial role in enabling developers to effectively manage and analyze the vast amounts of data generated by connected devices and sensors. By harnessing the combined power of Elastic and ROS, the possibilities for innovation in robotics are endless.
References
Mayr-Melnhof Group: Powering the Search for Real-Time Cost Savings
TRACTO maximizes construction equipment performance using Elastic Observability on Elastic Cloud
OTTO Motors: Using the Elastic Stack to Expand the IoT Landscape
Industrial Internet of Things (IIoT) with the Elastic Stack
From ELK Stack to Elastic Cloud Enterprise: Scaling Up Capabilities at John Deere
ROS: Market Outlook of Key Countries