Work with params and secrets
editWork with params and secretsedit
Params allow you to use dynamically defined values in your synthetic monitors. For example, you may want to test a production website with a particular demo account whose password is only known to the team managing the synthetic monitors.
For more information about security-sensitive use cases, refer to the documentation about sensitive values.
Define paramsedit
Param values can be declared by any of the following methods:
- In the Global parameters tab of the Synthetics Settings page in Kibana.
- Declaring a default value for the parameter in a configuration file.
-
Passing the
--params
CLI argument.
If you are creating and managing synthetic monitors using
projects, you can also use regular environment
variables via the standard node process.env
global object.
The values in the configuration file are read in the following order:
- Global parameters in Kibana: The Global parameters set in Kibana are read first.
- Configuration file: Then the Global parameters are merged with any parameters defined in a configuration file. If a parameter is defined in both Kibana and a configuration file, the value in the configuration file will be used.
-
CLI: Then the parameters defined in the configuration are merged with any parameters passed to the CLI
--params
argument. If a parameter is defined in a configuration file and using the CLI argument, the value defined using the CLI will be used. When running a script using the CLI, Kibana-defined Global parameters have no impact on the test because it won’t have access to Kibana.
Global parameters in Kibanaedit
In the Synthetics app:
- Go to Settings.
- Go to the Global parameters tab.
- Define parameters.
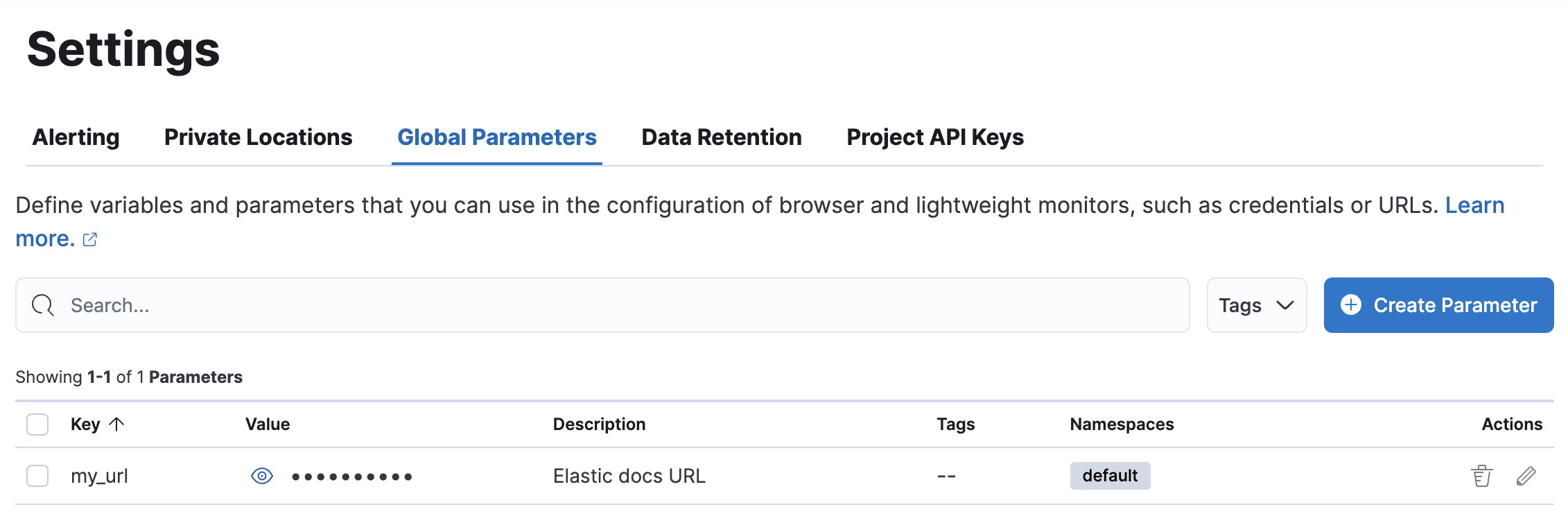
Project config fileedit
Use a synthetics.config.js
or synthetics.config.ts
file to define variables required by your tests.
This file should be placed in the root of your synthetics project.
export default (env) => { let my_url = "http://localhost:8080"; if (env === "production") { my_url = "https://elastic.github.io/synthetics-demo/" } return { params: { my_url, }, }; };
The example above uses the env
variable, which corresponds to the value of the NODE_ENV
environment variable.
CLI argumentedit
To set parameters when running npx @elastic/synthetics
on the command line,
use the --params
or -p
flag. The provided map is merged over any existing variables defined in the synthetics.config.{js,ts}
file.
For example, to override the my_url
parameter, you would run:
npx @elastic/synthetics . --params '{"my_url": "http://localhost:8080"}'
Use paramsedit
You can use params in both lightweight and browser monitors created in either a project or the Synthetics UI in Kibana.
In a projectedit
For lightweight monitors in projects, wrap the name of the param in ${}
(for example, ${my_url}
).
- type: http name: Todos Lightweight id: todos-lightweight urls: ["${my_url}"] schedule: '@every 1m'
In browser monitors, parameters can be referenced via the params
property available within the
argument to a journey
, before
, beforeAll
, after
, or afterAll
callback function.
Add params.
before the name of the param (for example, params.my_url
):
beforeAll(({params}) => { console.log(`Visiting ${params.my_url}`) }) journey("My Journey", ({ page, params }) => { step('launch app', async () => { await page.goto(params.my_url) }) })
In the UIedit
To use a param in a lightweight monitor that is created in the Synthetics UI,
wrap the name of the param in ${}
(for example, ${my_url}
).
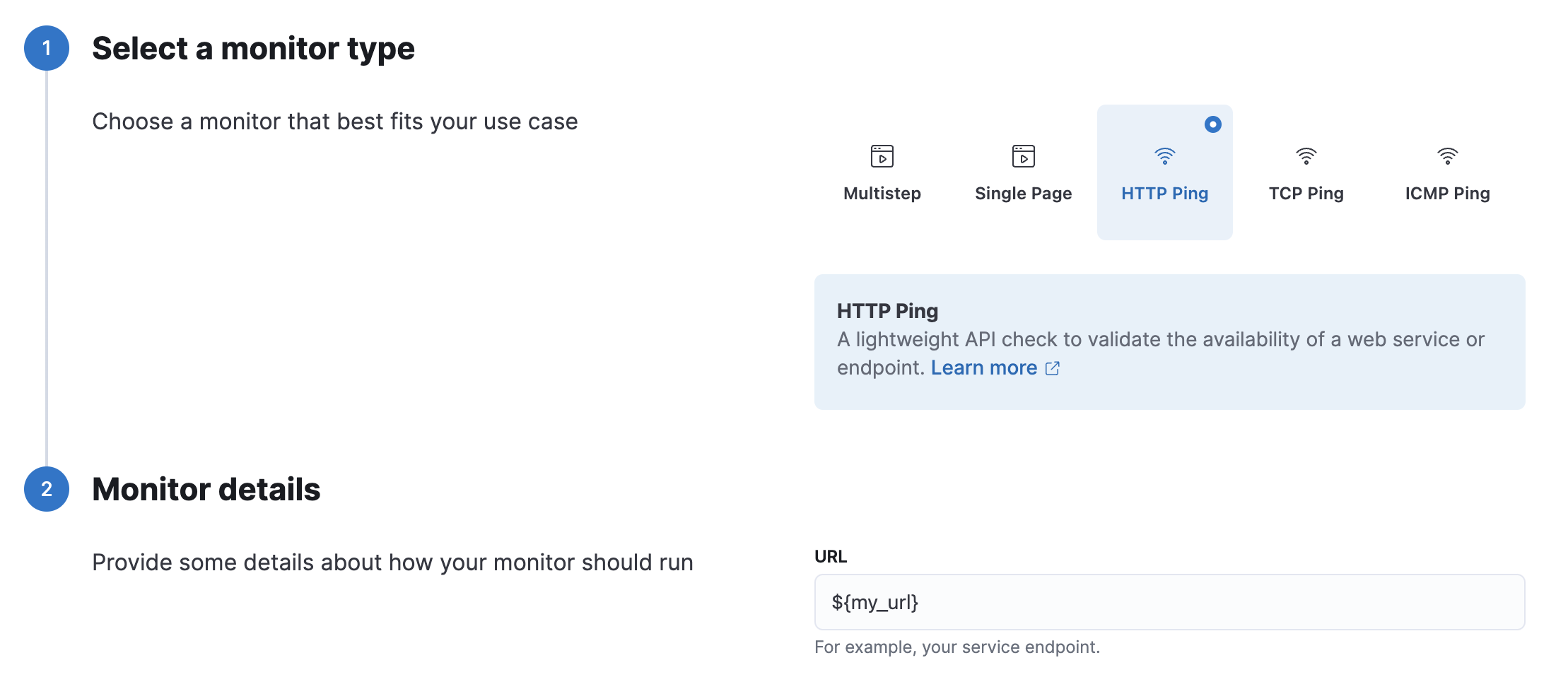
To use a param in a browser monitor that is created in the Synthetics UI,
add params.
before the name of the param (for example, params.my_url
).
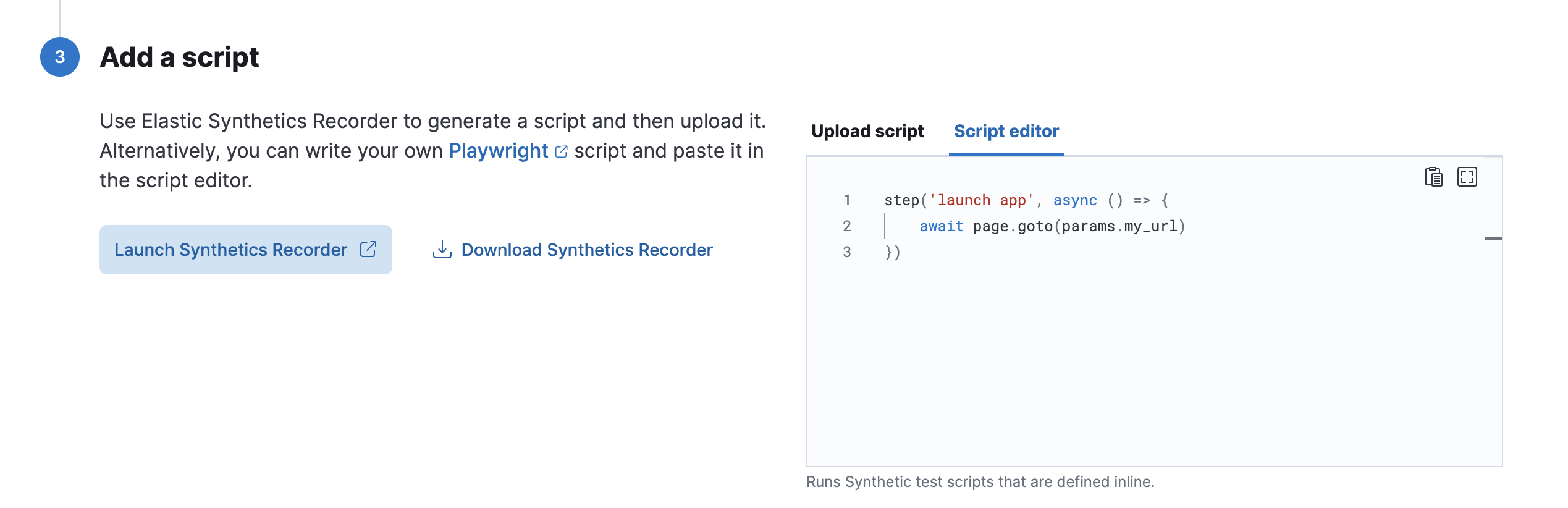
Working with secrets and sensitive valuesedit
Your synthetics scripts may require the use of passwords or other sensitive secrets that are not known until runtime.
Params are viewable in plain-text by administrators and other users with all
privileges for
the Synthetics app.
Also note that synthetics scripts have no limitations on accessing these values, and a malicious script author could write a
synthetics journey that exfiltrates params
and other data at runtime.
Do not to use truly sensitive passwords (for example, an admin password or a real credit card)
in any synthetics tools.
Instead, set up limited demo accounts, or fake credit cards with limited functionality.
If you want to limit access to parameters, ensure that users who are not supposed to access those values
do not have all
privileges for the Synthetics app, and that any scripts that use those values
do not leak them in network requests or screenshots.
If you are managing monitors with projects, you can use environment variables
in your synthetics.config.ts
or synthetics.config.js
file.
The example below uses process.env.MY_URL
to reference a variable named MY_URL
defined in the environment and assigns its value to a param. That param can then
be used in both lightweight and browser monitors that are managed in the project:
export default { params: { my_url: process.env.MY_URL } };