Connectingedit
This page contains the information you need to connect and use the Client with Elastic Enterprise Search.
On this page
Authenticationedit
This section contains code snippets to show you how to connect to Enterprise Search, App Search, and Workplace Search.
Each service has its own authentication schemes. Enterprise Search uses a basic HTTP authentication with username
and password
.
App Search and Workplace Search also accept a token
.
Refer to the authentication documentation for App Search and Workplace Search for the supported token types.
Authenticating with Enterprise Searchedit
Enterprise Search supports HTTP basic authentication with a username
and password
.
You can specify the enterprise-search
configuration in the Client
initialization:
use Elastic\EnterpriseSearch\Client; $client = new Client([ 'host' => 'https://id.ent-search.europe-west2.gcp.elastic-cloud.com', 'enterprise-search' => [ 'username' => '<insert here the username>', 'password' => '<insert here the password>' ] ]); $enterpriseSearch = $client->enterpriseSearch();
Or pass directly the username
and password
in the enterpriseSearch()
function:
use Elastic\EnterpriseSearch\Client; $client = new Client([ 'host' => 'https://id.ent-search.europe-west2.gcp.elastic-cloud.com', ]); $enterpriseSearch = $client->enterpriseSearch([ 'username' => '<insert here the username>', 'password' => '<insert here the password>' ]);
Authenticating with App Searchedit
Follow the App Search authentication guide to find or create an App Search API key.
The API key is the token
that you need to pass in the Client.
You can pass the token
in the Client
initialization:
use Elastic\EnterpriseSearch\Client; $client = new Client([ 'host' => 'https://id.ent-search.europe-west2.gcp.elastic-cloud.com', 'app-search' => [ 'token' => '<insert here the API key>' ] ]); $appSearch = $client->appSearch();
Or pass directly the token
in the appSearch()
function:
use Elastic\EnterpriseSearch\Client; $client = new Client([ 'host' => 'https://id.ent-search.europe-west2.gcp.elastic-cloud.com', ]); $appSearch = $client->appSearch([ 'token' => '<insert here the API key>' ]);
Authenticating with Workplace Searchedit
Workplace Search supports multiple authentication methods:
Workplace Search bearer tokensedit
Follow the Workplace Search API authentication reference to find or create a bearer token supported by Workplace Search.
You can pass the token
in the Client
initialization:
use Elastic\EnterpriseSearch\Client; $client = new Client([ 'host' => 'https://id.ent-search.europe-west2.gcp.elastic-cloud.com', 'workplace-search' => [ 'token' => '<insert here the access token>' ] ]); $workplaceSearch = $client->workplaceSearch();
Or pass directly the token
in the workplaceSearch()
function:
use Elastic\EnterpriseSearch\Client; $client = new Client([ 'host' => 'https://id.ent-search.europe-west2.gcp.elastic-cloud.com', ]); $workplaceSearch = $client->workplaceSearch([ 'token' => '<insert here the access token>' ]);
Elastic Cloudedit
If you want to use Elastic Cloud you have many options
to configure the enterprise-search-php
client.
The first settings is the host
domain to connect. You can copy the endpoint URL
from the Deployment page of the Elastic cloud dashboard:
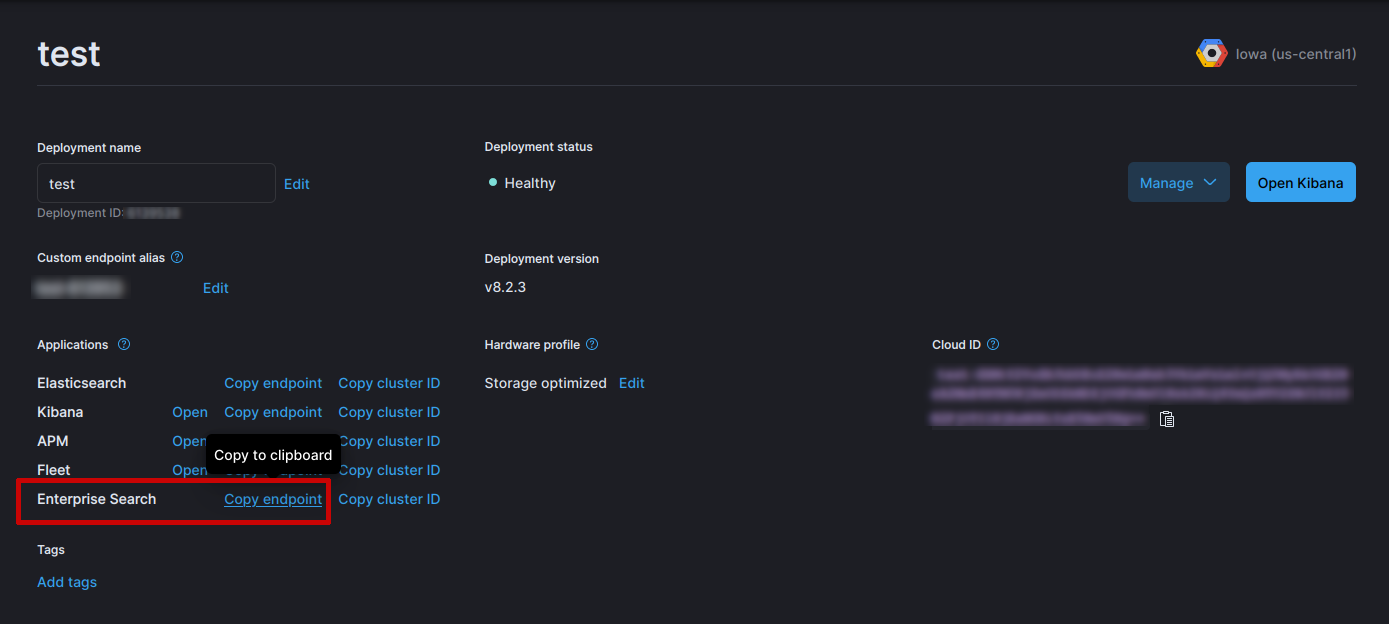
After, you need to choose the authentication mechanism for connecting to Enterprise Search, App Search and Workplace Search.
Authenticating with Enterprise Search in Cloudedit
You can use username (elastic
) and password (the one given during the deployment).
use Elastic\EnterpriseSearch\Client; $client = new Client([ 'host' => 'url/from/Elastic/Cloud/dashborad', 'enterprise-search' => [ 'username' => 'elastic' 'password' => 'the password from the deployment' ] ]); $es = $client->enterpriseSearch();
Or you can use the API Key created in the Management > API Keys.
use Elastic\EnterpriseSearch\Client; $client = new Client([ 'host' => 'url/from/Elastic/Cloud/dashborad', 'enterprise-search' => [ 'apiKey' => 'the API Key from Management' ] ]); $es = $client->enterpriseSearch();
Authenticating with App Search in Cloudedit
For App Search you can use different authentication mechanisms:
- username and password, as for Enteprise Search;
- Elasticsearch token;
- API key from the App Search Credentials.
use Elastic\EnterpriseSearch\Client; $client = new Client([ 'host' => 'url/from/Elastic/Cloud/dashborad', 'app-search' => [ 'username' => 'elastic' 'password' => 'the password from the deployment', // or 'token' => 'token created from Elasticsearch get token API', // or 'apiKey' => 'the API Key from App Search Credentials' ] ]); $app = $client->appSearch();
Authenticating with Workplace Search in Cloudedit
For Workplace Search you can use different authentication mechanisms:
- username and password, as for Enteprise Search;
- Elasticsearch token;
- Workplace Search API key
- Workplace Search OAuth token
use Elastic\EnterpriseSearch\Client; $client = new Client([ 'host' => 'url/from/Elastic/Cloud/dashborad', 'app-search' => [ 'username' => 'elastic' 'password' => 'the password from the deployment', // or 'token' => 'token created from Elasticsearch get token API', // or 'apiKey' => 'the API Key from Workplace Search API key' // or 'apiKey' => 'the token from Workplace Search OAuth' ] ]); $workplace = $client->workplaceSearch();