Create a first Deployment: Elasticsearch and Kibana
editCreate a first Deployment: Elasticsearch and Kibana
editFor our API exploration in some of the later examples, we need a basic deployment called My First Deployment
that includes an Elasticsearch cluster and a Kibana instance.
The following API call includes all the necessary JSON you need to create a deployment:
curl -k -X POST -H "Authorization: ApiKey $ECE_API_KEY" https://$COORDINATOR_HOST:12443/api/v1/deployments -H 'content-type: application/json' -d ' { "name": "My First Deployment", "resources": { "elasticsearch": [ { "ref_id": "main-elasticsearch", "region": "ece-region", "plan": { "cluster_topology": [ { "id": "hot_content", "node_roles": [ "master", "ingest", "transform", "data_hot", "remote_cluster_client", "data_content" ], "zone_count": 1, "elasticsearch": { "node_attributes": { "data": "hot" }, "enabled_built_in_plugins": [] }, "instance_configuration_id": "data.default", "size": { "value": 2048, "resource": "memory" } }, { "id": "warm", "node_roles": [ "data_warm", "remote_cluster_client" ], "zone_count": 1, "elasticsearch": { "node_attributes": { "data": "warm" }, "enabled_built_in_plugins": [] }, "instance_configuration_id": "data.highstorage", "size": { "value": 0, "resource": "memory" } }, { "id": "cold", "node_roles": [ "data_cold", "remote_cluster_client" ], "zone_count": 1, "elasticsearch": { "node_attributes": { "data": "cold" }, "enabled_built_in_plugins": [] }, "instance_configuration_id": "data.highstorage", "size": { "value": 0, "resource": "memory" } }, { "id": "frozen", "node_roles": [ "data_frozen" ], "zone_count": 1, "elasticsearch": { "node_attributes": { "data": "frozen" }, "enabled_built_in_plugins": [] }, "instance_configuration_id": "data.frozen", "size": { "value": 0, "resource": "memory" } }, { "id": "coordinating", "node_roles": [ "ingest", "remote_cluster_client" ], "zone_count": 1, "instance_configuration_id": "coordinating", "size": { "value": 0, "resource": "memory" }, "elasticsearch": { "enabled_built_in_plugins": [] } }, { "id": "master", "node_roles": [ "master", "remote_cluster_client" ], "zone_count": 1, "instance_configuration_id": "master", "size": { "value": 0, "resource": "memory" }, "elasticsearch": { "enabled_built_in_plugins": [] } }, { "id": "ml", "node_roles": [ "ml", "remote_cluster_client" ], "zone_count": 1, "instance_configuration_id": "ml", "size": { "value": 0, "resource": "memory" }, "elasticsearch": { "enabled_built_in_plugins": [] } } ], "elasticsearch": { "version": "8.13.2" }, "autoscaling_enabled": false, "deployment_template": { "id": "default" } }, "settings": { "dedicated_masters_threshold": 6, "snapshot": { "enabled": false } } } ], "kibana": [ { "ref_id": "main-kibana", "elasticsearch_cluster_ref_id": "main-elasticsearch", "region": "ece-region", "plan": { "zone_count": 1, "cluster_topology": [ { "instance_configuration_id": "kibana", "size": { "value": 1024, "resource": "memory" }, "zone_count": 1 } ], "kibana": { "version": "8.13.2" } } } ], "apm": [], "enterprise_search": [] } } '
-
resources
-
The set of resources in the deployment. In the JSON object, there are four resources in the deployment: one each of type
elasticsearch
,kibana
,apm
, andenterprise_search
. -
region
-
The region will always be
ece-region
, since this is currently the only region available in an ECE environment. -
ref_id
- The unique ID for a resource in a deployment. This is used to connect other resources or to obtain information about the resource. You can set the ID to any value.
-
deployment_template
-
The template used to create the deployment. In this case, the
default
template is used. Several other deployment templates are available and you can also create your own. -
ìnstance_configuration_id
- The instance configuration used for the node, indicating the default RAM and storage sizes. You can specify any default instance configuration or you can create your own.
-
node_roles
-
The roles assigned to each node in the deployment. Possible values are
master
,data_content
,data_hot
,data_warm
,data_cold
,data_frozen
,ingest
,ml
,remote_cluster_client
, andtransform
. For descriptions of these values, check Node roles. -
autoscaling_enabled
- Whether or not autoscaling is enabled for the deployment. For this basic example the deployment is created with autoscaling disabled, but for an example of using the API to set up a deployment with autoscaling configured, check Autoscaling through the API. To learn about the autoscaling feature itself, check Deployment autoscaling.
-
version
- The version of Elasticsearch and any other component to include in the deployment. You can create a component for any Elastic Stack version available in your ECE installation. You can also make other versions available by adding new Elastic Stack packs.
We use the same /api/v1/deployments
endpoint as in our first API example. The difference is that we now perform a POST request instead of a GET to create a deployment.
In the cluster_topology
section of the request, you’ll notice that the deployment includes one enabled Elasticsearch hot_content
tier with size set to 2048
, which allocates the node with 64GB of storage and 2GB of RAM. The deployment also includes warm
, cold
, and frozen
data tiers, as well as coordinating
, master
, and ml
nodes. However, since their size is set to 0
, they are are not enabled in the deployment when it is created. Check the Data tiers and Node roles documentation to learn more about these settings.
After the API call completes, ECE returns some information that you’re going to need later on, such as the deployment ID and the password for the elastic
superuser. Write this information down and keep it somewhere safe.
{ "id" : "$DEPLOYMENT_ID", "name" : "My First Deployment", "created" : true, "resources" : [ { "ref_id" : "main-elasticsearch", "id" : "$ELASTICSEARCH_CLUSTER_ID", "kind" : "elasticsearch", "region" : "ece-region", "cloud_id" : "My_First_Deployment:MTkyLjE2...kz", "credentials" : { "username" : "elastic", "password" : "$PASSWORD" } }, { "ref_id" : "main-kibana", "elasticsearch_cluster_ref_id" : "main-elasticsearch", "id" : "$KIBANA_ID", "kind" : "kibana", "region" : "ece-region" } ] }
Make sure to keep a record of the elastic
user’s password.
When the deployment setup is complete, Kibana will be available at the following URL: https://KIBANA_ID.ECE_DEPLOYMENT_DOMAIN_NAME:9243/
. When you navigate to Kibana from the ECE user console, you should be signed in directly to Kibana without needing to authenticate.
To find the ECE_DEPLOYMENT_DOMAIN_NAME run:
curl -k -X GET -H "Authorization: ApiKey $ECE_API_KEY" https://$COORDINATOR_HOST:12443/api/v1/platform/configuration/networking/deployment_domain_name
Use the elastic
user and returned random password when you first log in to Kibana.
Your newly created deployment is now accessible from the ECE UI:
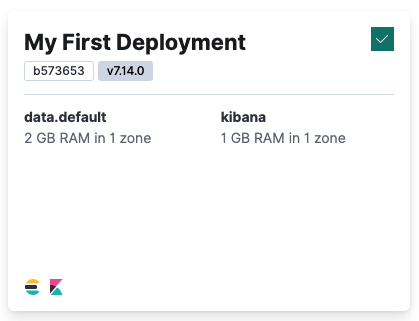