Getting started
editGetting startededit
This page guides you through the installation process of the Go client, shows you how to instantiate the client, and how to perform basic Elasticsearch operations with it. You can use the client with either a low-level API or a fully typed API. This getting started shows you examples of both APIs.
Requirementsedit
Go version 1.21+
Installationedit
To install the latest version of the client, run the following command:
go get github.com/elastic/go-elasticsearch/v8@latest
Refer to the Installation page to learn more.
Connectingedit
You can connect to the Elastic Cloud using an API key and the Elasticsearch endpoint for the low level API:
client, err := elasticsearch.NewClient(elasticsearch.Config{ CloudID: "<CloudID>", APIKey: "<ApiKey>", })
You can connect to the Elastic Cloud using an API key and the Elasticsearch endpoint for the fully-typed API:
typedClient, err := elasticsearch.NewTypedClient(elasticsearch.Config{ CloudID: "<CloudID>", APIKey: "<ApiKey>", })
Your Elasticsearch endpoint can be found on the My deployment page of your deployment:
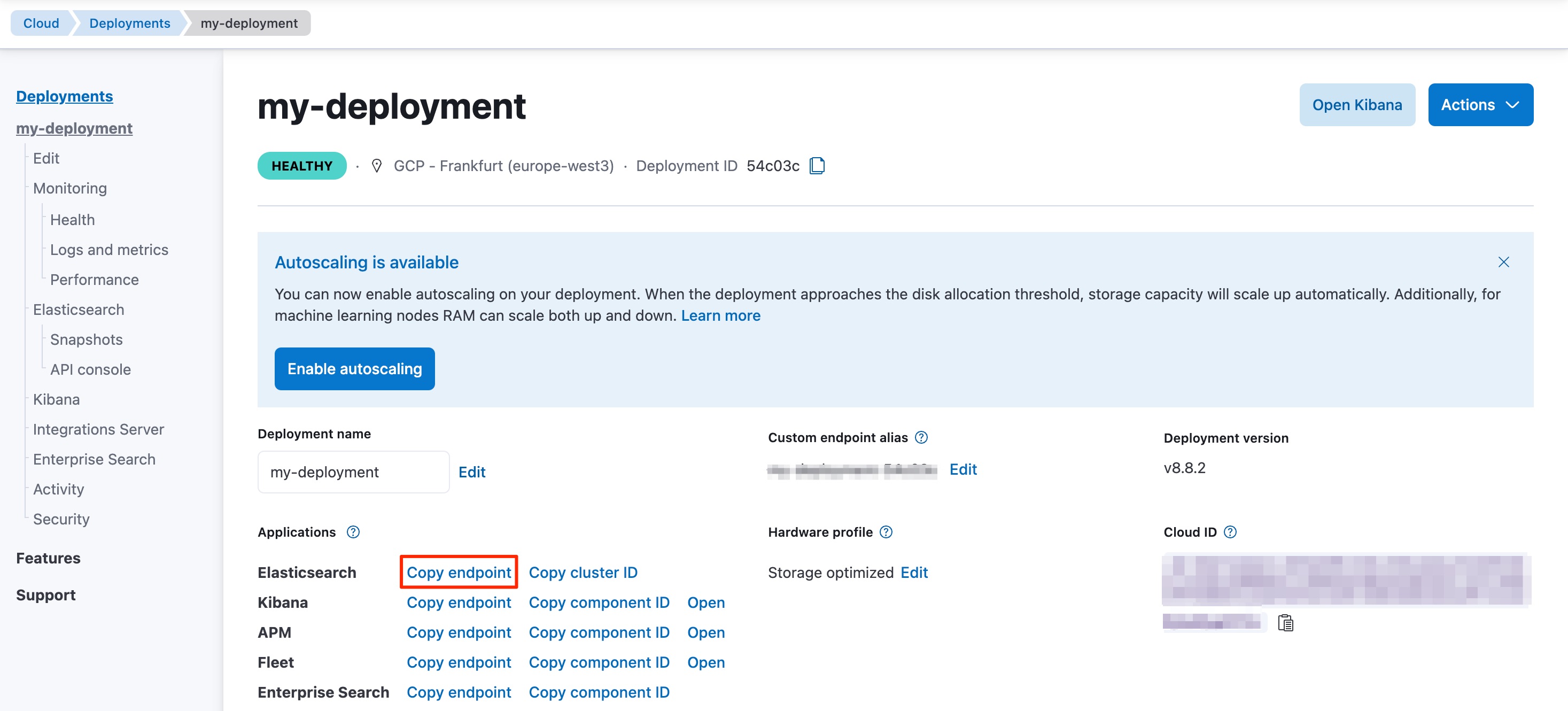
You can generate an API key on the Management page under Security.
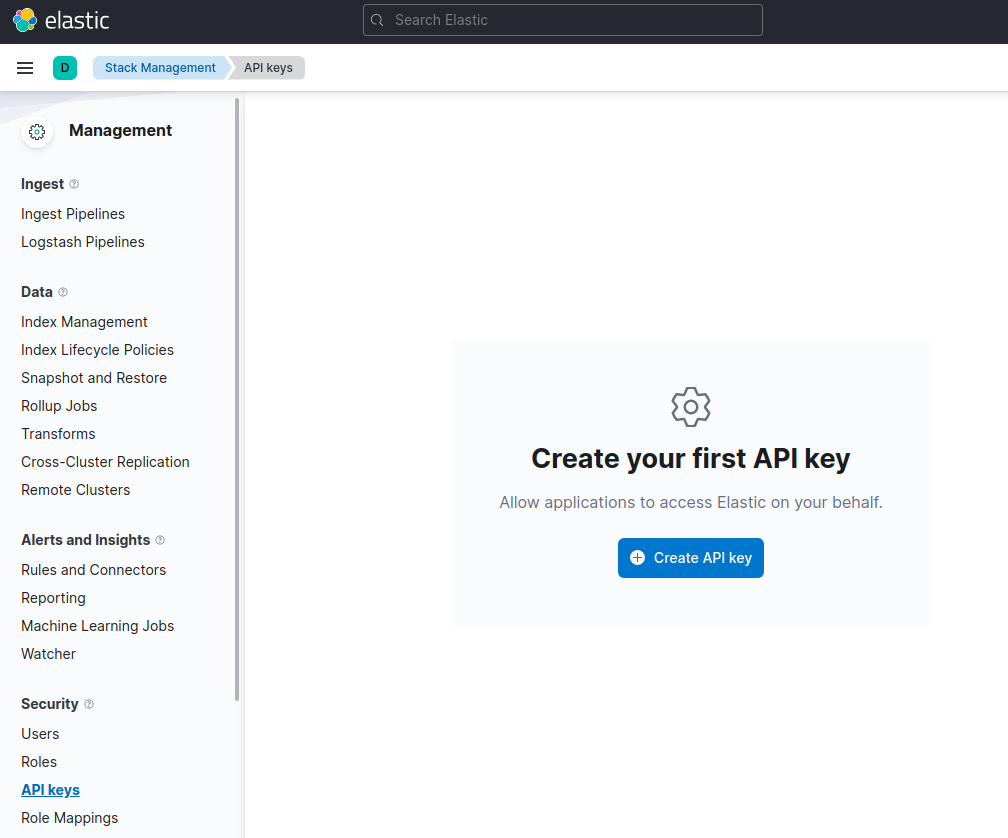
For other connection options, refer to the Connecting section.
Operationsedit
Time to use Elasticsearch! This section walks you through the basic, and most important, operations of Elasticsearch. For more operations and more advanced examples, refer to the Examples page.
Creating an indexedit
This is how you create the my_index
index with the low level API:
client.Indices.Create("my_index")
This is how you create the my_index
index with the fully-typed API:
typedClient.Indices.Create("my_index").Do(context.TODO())
Indexing documentsedit
This is a simple way of indexing a document by using the low-level API:
document := struct { Name string `json:"name"` }{ "go-elasticsearch", } data, _ := json.Marshal(document) client.Index("my_index", bytes.NewReader(data))
This is a simple way of indexing a document by using the fully-typed API:
document := struct { Name string `json:"name"` }{ "go-elasticsearch", } typedClient.Index("my_index"). Id("1"). Request(document). Do(context.TODO())
Getting documentsedit
You can get documents by using the following code with the low-level API:
client.Get("my_index", "id")
This is how you can get documents by using the fully-typed API:
typedClient.Get("my_index", "id").Do(context.TODO())
Searching documentsedit
This is how you can create a single match query with the low-level API:
query := `{ "query": { "match_all": {} } }` client.Search( client.Search.WithIndex("my_index"), client.Search.WithBody(strings.NewReader(query)), )
This is how you can perform a single match query with the fully-typed API:
typedClient.Search(). Index("my_index"). Request(&search.Request{ Query: &types.Query{MatchAll: &types.MatchAllQuery{}}, }). Do(context.TODO())
Updating documentsedit
This is how you can update a document, for example to add a new field, by using the low-level API:
client.Update("my_index", "id", strings.NewReader(`{doc: { language: "Go" }}`))
This is how you can update a document with the fully-typed API:
typedClient.Update("my_index", "id"). Request(&update.Request{ Doc: json.RawMessage(`{ language: "Go" }`), }).Do(context.TODO())
Deleting documentsedit
client.Delete("my_index", "id")
typedClient.Delete("my_index", "id").Do(context.TODO())
Deleting an indexedit
client.Indices.Delete([]string{"my_index"})
typedClient.Indices.Delete("my_index").Do(context.TODO())
Further readingedit
- Learn more about the Typed API, a strongly typed Golang API for Elasticsearch.