Semantic searchedit
Semantic search is a search method that helps you find data based on the intent and contextual meaning of a search query, instead of a match on query terms (lexical search).
Elasticsearch provides semantic search capabilities using natural language processing (NLP) and vector search. Deploying an NLP model to Elasticsearch enables it to extract text embeddings out of text. Embeddings are vectors that provide a numeric representation of a text. Pieces of content with similar meaning have similar representations.
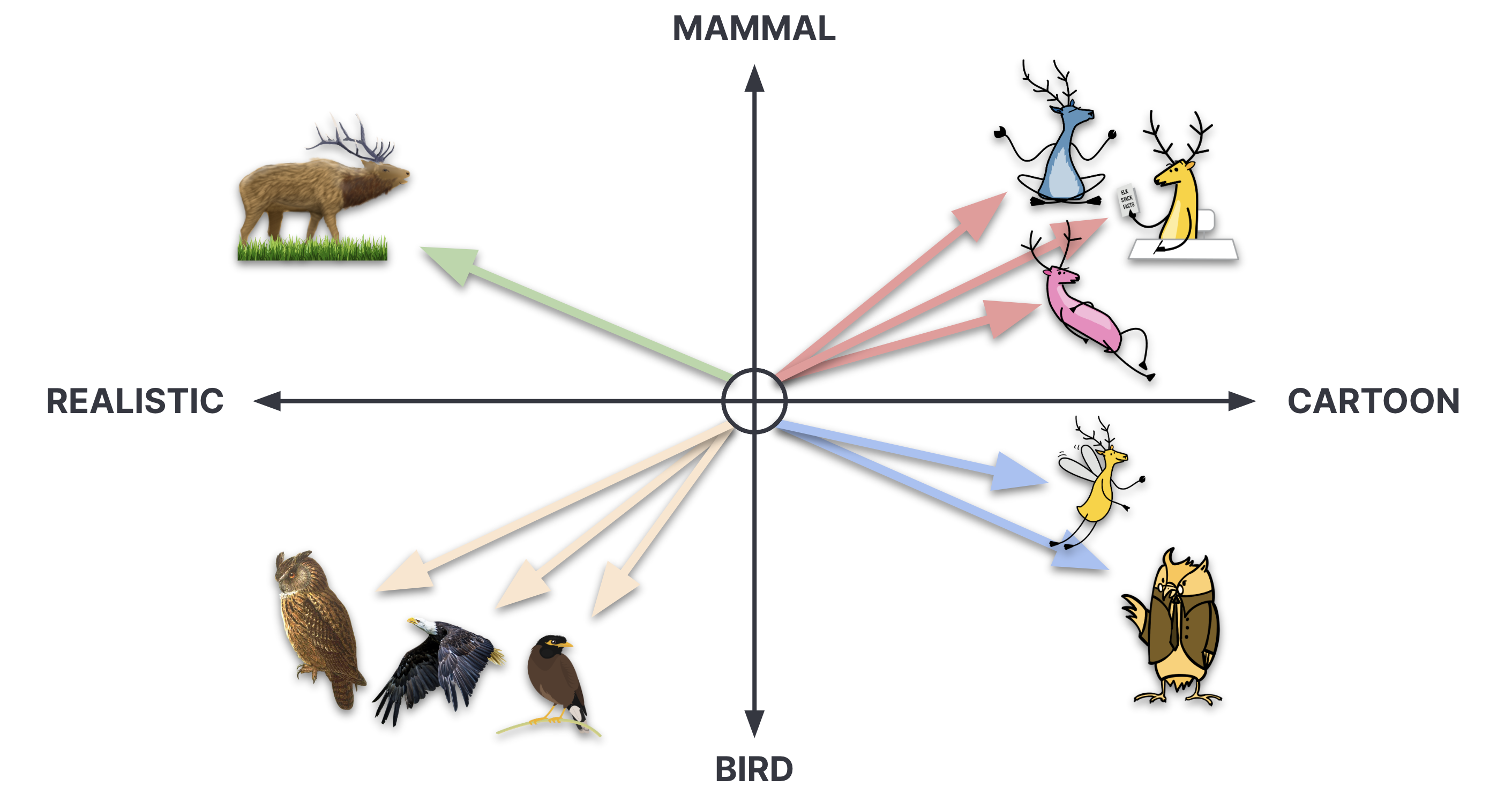
At query time, Elasticsearch can use the same NLP model to convert a query into embeddings, enabling you to find documents with similar text embeddings.
This guide shows you how to implement semantic search with Elasticsearch, from selecting an NLP model, to writing queries.
Select an NLP modeledit
Elasticsearch offers the usage of a wide range of NLP models, including both dense and sparse vector models. Your choice of the language model is critical for implementing semantic search successfully.
While it is possible to bring your own text embedding model, achieving good search results through model tuning is challenging. Selecting an appropriate model from our third-party model list is the first step. Training the model on your own data is essential to ensure better search results than using only BM25. However, the model training process requires a team of data scientists and ML experts, making it expensive and time-consuming.
To address this issue, Elastic provides a pre-trained representational model called Elastic Learned Sparse EncodeR (ELSER). ELSER, currently available only for English, is an out-of-domain sparse vector model that does not require fine-tuning. This adaptability makes it suitable for various NLP use cases out of the box. Unless you have a team of ML specialists, it is highly recommended to use the ELSER model.
In the case of sparse vector representation, the vectors mostly consist of zero values, with only a small subset containing non-zero values. This representation is commonly used for textual data. In the case of ELSER, each document in an index and the query text itself are represented by high-dimensional sparse vectors. Each non-zero element of the vector corresponds to a term in the model vocabulary. The ELSER vocabulary contains around 30000 terms, so the sparse vectors created by ELSER contain about 30000 values, the majority of which are zero. Effectively the ELSER model is replacing the terms in the original query with other terms that have been learnt to exist in the documents that best match the original search terms in a training dataset, and weights to control how important each is.
Deploy the modeledit
After you decide which model you want to use for implementing semantic search, you need to deploy the model in Elasticsearch.
To deploy ELSER, refer to Download and deploy ELSER.
To deploy a third-party text embedding model, refer to Deploy a text embedding model.
Map a field for the text embeddingsedit
Before you start using the deployed model to generate embeddings based on your input text, you need to prepare your index mapping first. The mapping of the index depends on the type of model.
ELSER produces token-weight pairs as output from the input text and the query.
The Elasticsearch sparse_vector
field type can store these
token-weight pairs as numeric feature vectors. The index must have a field with
the sparse_vector
field type to index the tokens that ELSER generates.
To create a mapping for your ELSER index, refer to the
Create the index mapping section of the tutorial. The example
shows how to create an index mapping for my-index
that defines the
my_embeddings.tokens
field - which will contain the ELSER output - as a
sparse_vector
field.
response = client.indices.create( index: 'my-index', body: { mappings: { properties: { my_tokens: { type: 'sparse_vector' }, my_text_field: { type: 'text' } } } } ) puts response
PUT my-index { "mappings": { "properties": { "my_tokens": { "type": "sparse_vector" }, "my_text_field": { "type": "text" } } } }
The name of the field that will contain the tokens generated by ELSER. |
|
The field that contains the tokens must be a |
|
The name of the field from which to create the sparse vector representation.
In this example, the name of the field is |
|
The field type is |
The models compatible with Elasticsearch NLP generate dense vectors as output. The
dense_vector
field type is suitable for storing dense vectors
of numeric values. The index must have a field with the dense_vector
field
type to index the embeddings that the supported third-party model that you
selected generates. Keep in mind that the model produces embeddings with a
certain number of dimensions. The dense_vector
field must be configured with
the same number of dimensions using the dims
option. Refer to the respective
model documentation to get information about the number of dimensions of the
embeddings.
To review a mapping of an index for an NLP model, refer to the mapping code
snippet in the
Add the text embedding model to an ingest inference pipeline
section of the tutorial. The example shows how to create an index mapping that
defines the my_embeddings.predicted_value
field - which will contain the model
output - as a dense_vector
field.
response = client.indices.create( index: 'my-index', body: { mappings: { properties: { 'my_embeddings.predicted_value' => { type: 'dense_vector', dims: 384 }, my_text_field: { type: 'text' } } } } ) puts response
PUT my-index { "mappings": { "properties": { "my_embeddings.predicted_value": { "type": "dense_vector", "dims": 384 }, "my_text_field": { "type": "text" } } } }
The name of the field that will contain the embeddings generated by the model. |
|
The field that contains the embeddings must be a |
|
The model produces embeddings with a certain number of dimensions. The
|
|
The name of the field from which to create the dense vector representation.
In this example, the name of the field is |
|
The field type is |
Generate text embeddingsedit
Once you have created the mappings for the index, you can generate text embeddings from your input text. This can be done by using an ingest pipeline with an inference processor. The ingest pipeline processes the input data and indexes it into the destination index. At index time, the inference ingest processor uses the trained model to infer against the data ingested through the pipeline. After you created the ingest pipeline with the inference processor, you can ingest your data through it to generate the model output.
This is how an ingest pipeline that uses the ELSER model is created:
response = client.ingest.put_pipeline( id: 'my-text-embeddings-pipeline', body: { description: 'Text embedding pipeline', processors: [ { inference: { model_id: '.elser_model_2', input_output: [ { input_field: 'my_text_field', output_field: 'my_tokens' } ] } } ] } ) puts response
PUT _ingest/pipeline/my-text-embeddings-pipeline { "description": "Text embedding pipeline", "processors": [ { "inference": { "model_id": ".elser_model_2", "input_output": [ { "input_field": "my_text_field", "output_field": "my_tokens" } ] } } ] }
Configuration object that defines the |
To ingest data through the pipeline to generate tokens with ELSER, refer to the Ingest the data through the inference ingest pipeline section of the tutorial. After you successfully ingested documents by using the pipeline, your index will contain the tokens generated by ELSER. Tokens are learned associations capturing relevance, they are not synonyms. To learn more about what tokens are, refer to this page.
This is how an ingest pipeline that uses a text embedding model is created:
response = client.ingest.put_pipeline( id: 'my-text-embeddings-pipeline', body: { description: 'Text embedding pipeline', processors: [ { inference: { model_id: 'sentence-transformers__msmarco-minilm-l-12-v3', target_field: 'my_embeddings', field_map: { my_text_field: 'text_field' } } } ] } ) puts response
PUT _ingest/pipeline/my-text-embeddings-pipeline { "description": "Text embedding pipeline", "processors": [ { "inference": { "model_id": "sentence-transformers__msmarco-minilm-l-12-v3", "target_field": "my_embeddings", "field_map": { "my_text_field": "text_field" } } } ] }
The model ID of the text embedding model you want to use. |
|
The |
To ingest data through the pipeline to generate text embeddings with your chosen model, refer to the Add the text embedding model to an inference ingest pipeline section. The example shows how to create the pipeline with the inference processor and reindex your data through the pipeline. After you successfully ingested documents by using the pipeline, your index will contain the text embeddings generated by the model.
Now it is time to perform semantic search!
Search the dataedit
Depending on the type of model you have deployed, you can query rank features with a text expansion query, or dense vectors with a kNN search.
ELSER text embeddings can be queried using a text expansion query. The text expansion query enables you to query a rank features field or a sparse vector field, by providing the model ID of the NLP model, and the query text:
response = client.search( index: 'my-index', body: { query: { text_expansion: { my_tokens: { model_id: '.elser_model_2', model_text: 'the query string' } } } } ) puts response
Text embeddings produced by dense vector models can be queried using a
kNN search. In the knn
clause, provide the name of the
dense vector field, and a query_vector_builder
clause with the model ID and
the query text.
response = client.search( index: 'my-index', body: { knn: { field: 'my_embeddings.predicted_value', k: 10, num_candidates: 100, query_vector_builder: { text_embedding: { model_id: 'sentence-transformers__msmarco-minilm-l-12-v3', model_text: 'the query string' } } } } ) puts response
GET my-index/_search { "knn": { "field": "my_embeddings.predicted_value", "k": 10, "num_candidates": 100, "query_vector_builder": { "text_embedding": { "model_id": "sentence-transformers__msmarco-minilm-l-12-v3", "model_text": "the query string" } } } }
Beyond semantic search with hybrid searchedit
In some situations, lexical search may perform better than semantic search. For example, when searching for single words or IDs, like product numbers.
Combining semantic and lexical search into one hybrid search request using reciprocal rank fusion provides the best of both worlds. Not only that, but hybrid search using reciprocal rank fusion has been shown to perform better in general.
Hybrid search between a semantic and lexical query can be achieved by using a
sub_searches
clause in your search request. In the sub_searches
clause,
provide a text_expansion
query and a full-text query. Next to the
sub_searches
clause, also provide a rank
clause with
the rrf
parameter to rank documents using reciprocal rank fusion.
GET my-index/_search { "sub_searches": [ { "query": { "match": { "my_text_field": "the query string" } } }, { "query": { "text_expansion": { "my_tokens": { "model_id": ".elser_model_2", "model_text": "the query string" } } } } ], "rank": { "rrf": {} } }
Hybrid search between a semantic and lexical query can be achieved by providing:
-
a
query
clause for the full-text query; -
a
knn
clause with the kNN search that queries the dense vector field; -
and a
rank
clause with therrf
parameter to rank documents using reciprocal rank fusion.
GET my-index/_search { "query": { "match": { "my_text_field": "the query string" } }, "knn": { "field": "text_embedding.predicted_value", "k": 10, "num_candidates": 100, "query_vector_builder": { "text_embedding": { "model_id": "sentence-transformers__msmarco-minilm-l-12-v3", "model_text": "the query string" } } }, "rank": { "rrf": {} } }
Read moreedit
-
Tutorials:
-
Blogs:
-
Interactive examples:
-
The
elasticsearch-labs
repo contains a number of interactive semantic search examples in the form of executable Python notebooks, using the Elasticsearch Python client
-
The